Python’s recursion allows us to solve complex problems by breaking them down into simpler subproblems. In this illustration, we’ll delve into a Python program that employs recursion to determine the total sum of a nested list.
Problem Statement
Develop a Python program that calculates and presents the total sum of a given nested list. The program should use recursion to navigate through the nested structure, adding up all the individual elements, regardless of the depth of nesting.
Python Program to Find the Total Sum of a Nested List Using Recursion
def nested_list_sum(nested_list): total_sum = 0 for element in nested_list: if isinstance(element, list): total_sum += nested_list_sum(element) else: total_sum += element return total_sum # Input and Output sample_nested_list = [1, [2, 3, [4, 5]], [6, [7]]] total = nested_list_sum(sample_nested_list) print("Nested List:", sample_nested_list) print("Total Sum:", total)
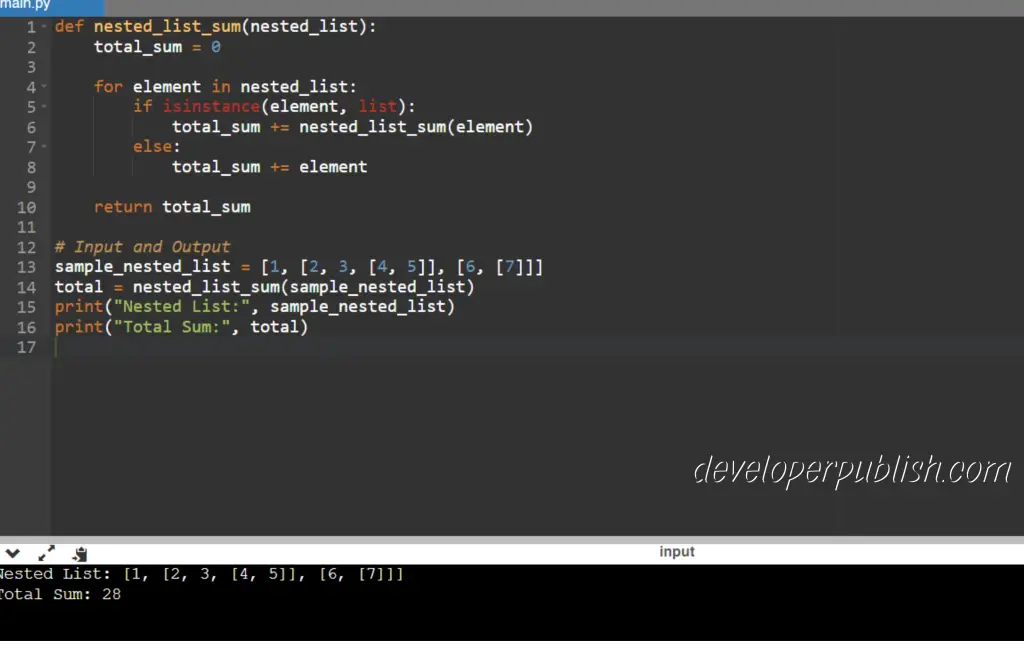