In this Python program, we’ll tackle the task of finding the largest element within a Doubly Linked List. Doubly Linked Lists are versatile data structures, and by solving this problem, you can enhance your understanding of working with them.
Problem Statement
Given a Doubly Linked List, the goal is to find and output the largest element present in it.
Python Program to Find the Largest Element in a Doubly Linked List
class Node: def __init__(self, data): self.data = data self.prev = None self.next = None class DoublyLinkedList: def __init__(self): self.head = None def insert(self, data): new_node = Node(data) if not self.head: self.head = new_node else: current = self.head while current.next: current = current.next current.next = new_node new_node.prev = current def find_largest_element(self): if not self.head: return None current = self.head largest = current.data while current: if current.data > largest: largest = current.data current = current.next return largest # Example usage if __name__ == "__main__": dll = DoublyLinkedList() elements = [12, 45, 7, 23, 56, 34] for element in elements: dll.insert(element) largest = dll.find_largest_element() print("Largest element:", largest)
Input / Output
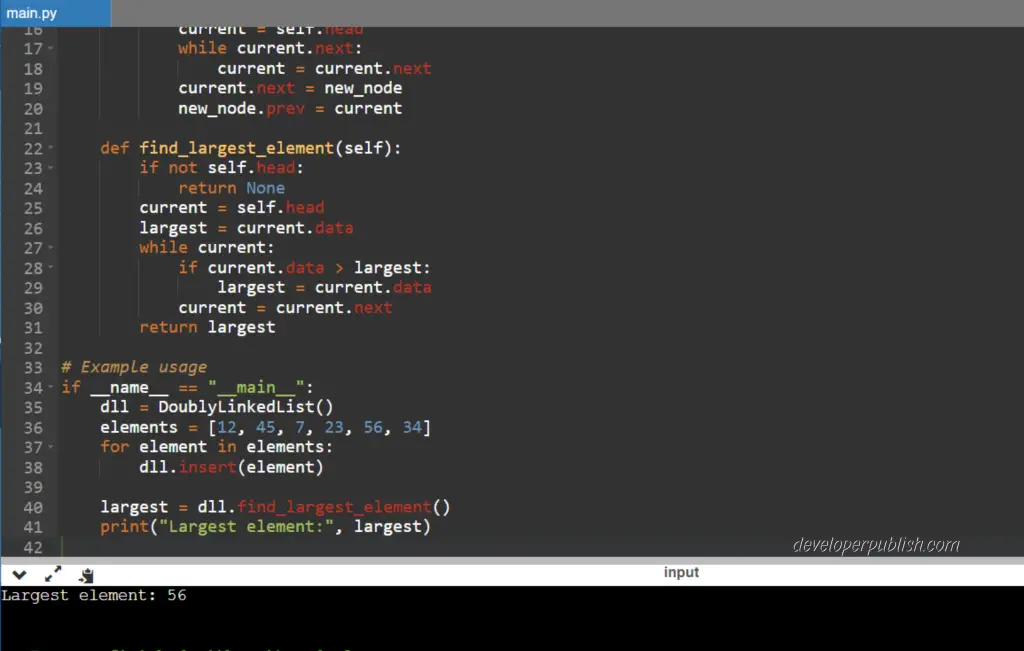