This Python program counts the occurrences of a specified word in a text file
Problem Statement
You are tasked with creating a Python program that reads the content of a given text file and counts the occurrences of a specific target word. Your program should provide the user with a way to input the target word, and it should then display the count of how many times that word appears in the text file, regardless of its capitalization.
Python Program to Count the Occurrences of a Word in a Text File
def count_word_occurrences(file_path, target_word): try: with open(file_path, 'r') as file: content = file.read() word_count = content.lower().count(target_word.lower()) return word_count except FileNotFoundError: print("File not found.") return 0 file_path = 'path/to/your/text/file.txt' # Update this with the actual file path target_word = input("Enter the word to count: ") occurrences = count_word_occurrences(file_path, target_word) print(f"The word '{target_word}' appears {occurrences} times in the file.")
How it Works
1. Defining the Function count_word_occurrences
This function takes two parameters: file_path
(the path to the text file) and target_word
(the word to be counted). Inside the function:
- The
try
block attempts to open the specified file in read mode using awith
statement. This is a context manager that ensures the file is properly closed after its suite finishes executing. - If the file is successfully opened, its content is read using the
read()
method, and the lowercase version of the content is stored in thecontent
variable. - The
count()
method is used to count the occurrences of the lowercasetarget_word
within the lowercasecontent
. - The function returns the count of occurrences.
If a FileNotFoundError
occurs (i.e., the specified file doesn’t exist), the except
block prints an error message and returns 0
.
2. Getting User Input and Counting Occurrences
- The
file_path
variable is set to the path of the text file you want to process. Replace'path/to/your/text/file.txt'
with the actual path. - The
target_word
variable is assigned the word entered by the user using theinput()
function. - The
count_word_occurrences
function is called with thefile_path
andtarget_word
as arguments, and the result is stored in theoccurrences
variable. - Finally, the program prints the result, showing how many times the
target_word
appears in the file.
3. Case Insensitivity
Both the content of the file and the target word are converted to lowercase using the lower()
method. This ensures that the search is case-insensitive. For example, if the target word is “Python” and the file contains “python” or “PYTHON,” they will all be counted as occurrences.
Input/ Output
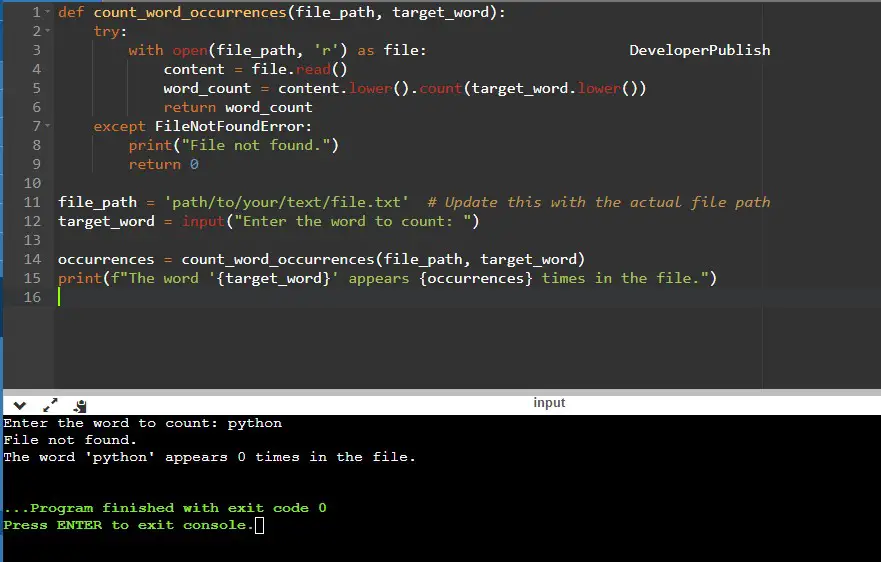