A perfect number is a positive integer that is equal to the sum of its proper divisors, excluding itself. For example, the number 28 is a perfect number because its divisors (excluding itself) are 1, 2, 4, 7, and 14, and their sum is 28.
Here’s a Python program to check whether a given number is a perfect number:
Problem Statement
Write a Python program that checks whether a given number is a perfect number or not. A perfect number is a positive integer that is equal to the sum of its proper divisors (excluding itself).
Your program should include the following:
- A function named
is_perfect_number
that takes an integernumber
as input. - The function should return
True
if the number is a perfect number, andFalse
otherwise. - Proper divisors are the positive divisors of a number excluding the number itself.
- The program should prompt the user to enter a number.
- After receiving the input, the program should call the
is_perfect_number
function to check if the number is perfect. - Finally, the program should display an appropriate message indicating whether the number is perfect or not.
Python Program to Check Whether a Given Number is Perfect Number
def is_perfect_number(number): sum_of_divisors = 0 for i in range(1, number): if number % i == 0: sum_of_divisors += i return sum_of_divisors == number # Example usage num = int(input("Enter a number: ")) if is_perfect_number(num): print(num, "is a perfect number.") else: print(num, "is not a perfect number.")
How it Works
- The program starts by defining a function called
is_perfect_number
that takes an integernumber
as input. This function will determine whether the given number is a perfect number or not. - Within the
is_perfect_number
function, a variable calledsum_of_divisors
is initialized to 0. This variable will store the sum of the proper divisors of the number. - A
for
loop is used to iterate over the range from 1 tonumber - 1
. This loop will check each number as a potential divisor of the given number. - Inside the loop, an
if
statement checks if the current number is a divisor ofnumber
. This is done by checking ifnumber
moduloi
equals 0. If it does, theni
is a divisor. - If
i
is a divisor, it is added to thesum_of_divisors
using the+=
operator. - After the loop completes, the
sum_of_divisors
is compared with the original number. If they are equal, it means the number is a perfect number, and the function returnsTrue
. - If the
sum_of_divisors
is not equal to the number, the function returnsFalse
, indicating that the number is not perfect. - In the main part of the program, the user is prompted to enter a number using the
input()
function. - The input is then converted to an integer using the
int()
function and stored in thenum
variable. - The
is_perfect_number
function is called with thenum
variable as an argument to check whether it is a perfect number or not. - Depending on the returned value from the
is_perfect_number
function, an appropriate message is displayed using theprint()
function to indicate whether the number is perfect or not. - The program terminates after displaying the result for one number.
That’s how the program works! It calculates the sum of proper divisors and compares it with the original number to determine if it is a perfect number.
Input/ Output
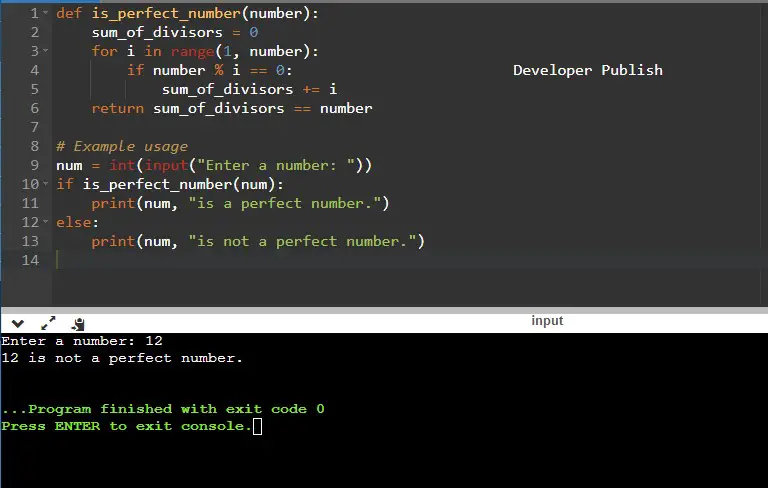
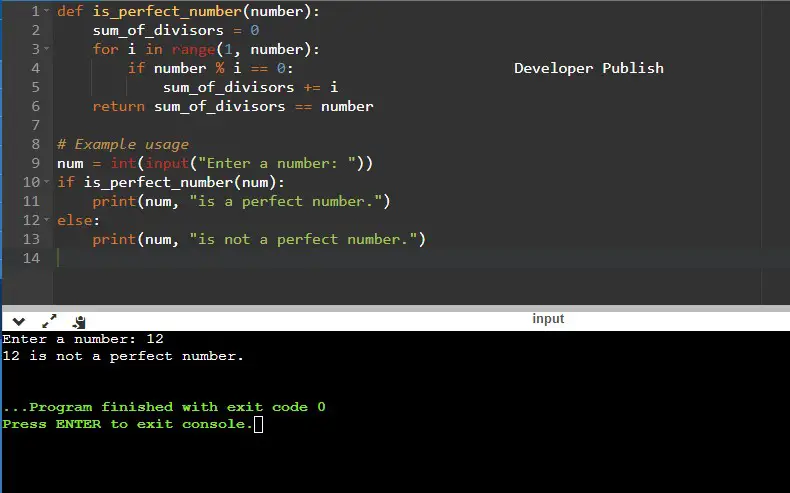