This C program finds the next lower power of 2 for a given integer using bitwise operations.
Problem statement
Given an integer num
, we need to find the next lower power of 2 (round floor) for this integer. The program should use bitwise operations to efficiently perform the calculation and display the result.
C Program to Round Floor of Integer to Next Lower Power of 2
#include <stdio.h> int roundFloorToPowerOf2(int num) { if (num < 2) return 0; int power = 1; while (power <= num / 2) { power *= 2; } return power; } int main() { int num; printf("Enter an integer: "); scanf("%d", &num); int result = roundFloorToPowerOf2(num); printf("The floor of %d to the next lower power of 2 is %d\n", num, result); return 0; }
How it Works
- The function takes an integer
num
as input. - If the input number
num
is less than 2, the function returns 0. This is because there is no lower power of 2 to round down to when the input is 0 or 1. - If the input number
num
is greater than or equal to 2, the function initializes a variablepower
to 1. This variable will be used to track the power of 2. - The function enters a loop that continues as long as
power
is less than or equal to half of the input number (num / 2
). - Inside the loop, the
power
variable is doubled in each iteration using the statementpower *= 2
. This ensures thatpower
represents the largest power of 2 that is less than or equal to the input number. - Once the loop terminates, the function has found the next lower power of 2 for the input number. It returns the value of
power
.
In the main
function, the user is prompted to enter an integer, and the input is stored in the num
variable. Then, the roundFloorToPowerOf2
function is called with num
as the argument. The returned value is stored in the result
variable.
Finally, the program displays the result on the console using printf
.
Input / Output
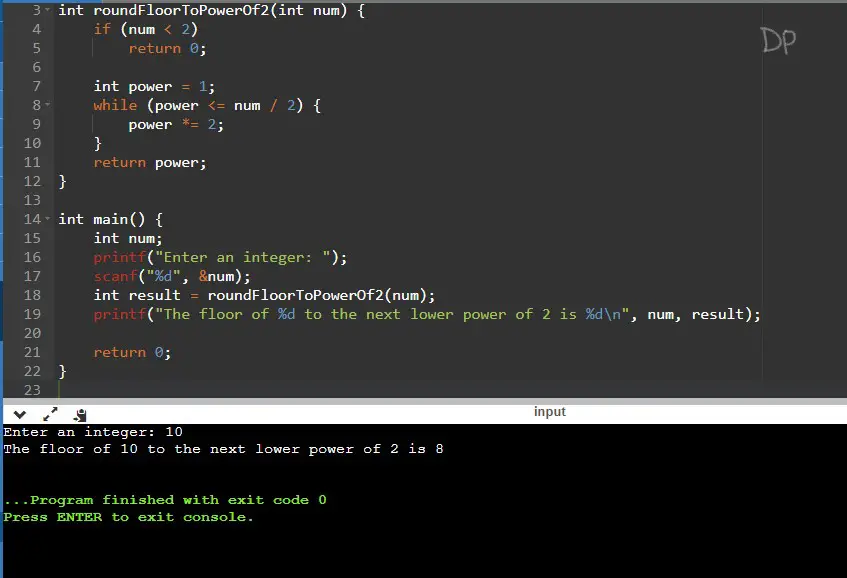