In this C program, we will write a function to increment each digit of a given integer by 1. We will take an integer as input from the user and then increment each digit of that integer by 1. The modified number will be displayed as the output.
Problem Statement
Given an integer, we need to increment each of its digits by 1 and display the modified number.
C Program to Increment by 1 to all the Digits of a Given Integer
#include <stdio.h> int incrementDigits(int num); int main() { int number; printf("Enter an integer: "); scanf("%d", &number); int result = incrementDigits(number); printf("Modified number: %d\n", result); return 0; } int incrementDigits(int num) { int modifiedNum = 0; int multiplier = 1; while (num != 0) { int digit = num % 10; digit = (digit + 1) % 10; modifiedNum += digit * multiplier; multiplier *= 10; num /= 10; } return modifiedNum; }
How it Works?
- The program defines a function
incrementDigits
that takes an integernum
as input and returns the modified number where each digit is incremented by 1. - In the
main
function, we prompt the user to enter an integer and store it in the variablenumber
. - We then call the
incrementDigits
function, passing thenumber
as an argument, and store the returned modified number in the variableresult
. - Finally, we display the modified number by printing the value of
result
.
Input/Output
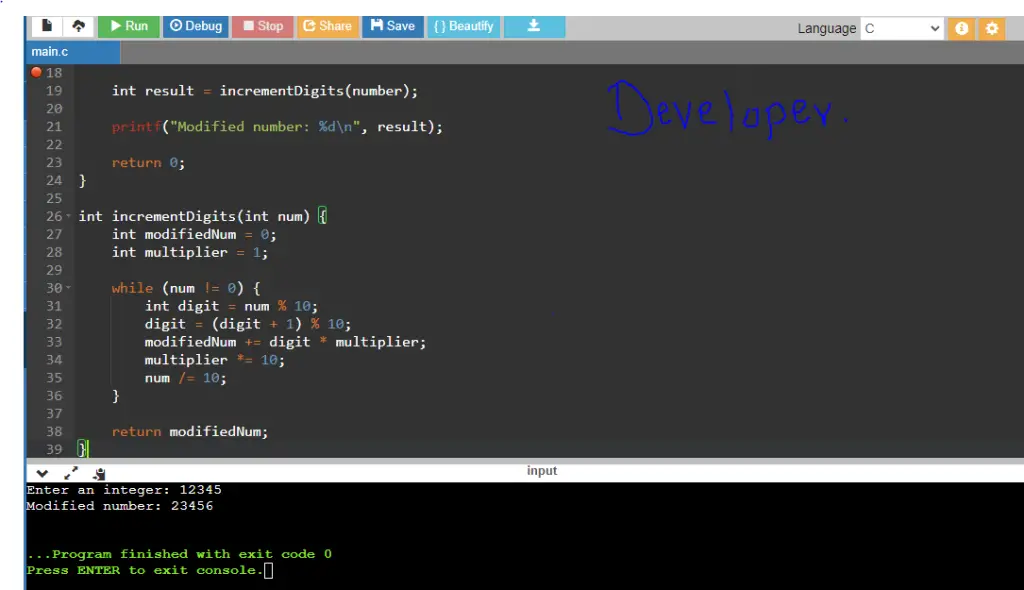