The strpbrk()
function is a standard library function in the C programming language that is used to search for the first occurrence of any character from a given set of characters in a string. It is declared in the <string.h>
header file.
The function signature of strpbrk()
is:
char *strpbrk(const char *str1, const char *str2);
Here, str1
is the string in which the search is performed, and str2
is the set of characters to be matched. The function returns a pointer to the first occurrence of any character from str2
in str1
or NULL
if no matching characters are found.
The strpbrk()
function performs a linear search through str1
, examining each character one by one. It compares each character in str1
with each character in str2
until a match is found. If a match is found, the function returns a pointer to the matching character in str1
. The search stops as soon as a match is found, so it does not necessarily return the first occurrence of the first character in str2
.
The strpbrk()
function is useful when you want to determine if a string contains any of the characters from a given set of characters. It provides a simple way to search for a group of characters within a string and can be used in various applications, such as string manipulation, parsing, and pattern matching.
Problem statement
You are given two strings, str1
and str2
. Your task is to implement the strpbrk()
function, which searches str1
for the first occurrence of any character from str2
and returns a pointer to the matching character in str1
. If no matching characters are found, the function should return NULL
.
Write a C function with the following signature:
Input:
- The function takes two arguments:
str1
(const char*): A null-terminated string in which the search is performed.str2
(const char*): A null-terminated string containing the set of characters to be matched.
Output:
- The function should return a pointer to the first occurrence of any character from
str2
instr1
, orNULL
if no matching characters are found.
Constraints:
- The input strings
str1
andstr2
consist of printable ASCII characters (excluding null characters). - The length of
str1
andstr2
is at most 10^5.
Note:
- The search should stop as soon as a matching character is found. The returned pointer should point to the first matching character in
str1
. - If
str1
orstr2
is an empty string (length 0), the function should returnNULL
. - If
str1
isNULL
orstr2
isNULL
, the function should returnNULL
.
Implement the strpbrk()
function and write a sample C program to demonstrate its usage
C Program to Implement strpbrk() Function
#include <stdio.h> #include <string.h> int main() { char str1[] = "Hello, world!"; char str2[] = "o,ld"; char *result = strpbrk(str1, str2); if (result != NULL) { printf("First matching character found: %c\n", *result); } else { printf("No matching characters found.\n"); } return 0; }
How it Works?
The strpbrk()
function works by performing a linear search through the string str1
and comparing each character with each character in the string str2
. Here’s how the function works:
- The function takes two arguments:
str1
andstr2
. - It initializes two pointers:
p1
pointing to the start ofstr1
, andp2
that will be used for iterating throughstr2
. - The function enters a loop that continues until the end of
str1
is reached (i.e., until the character pointed byp1
is not null). - Inside the loop, the function enters another loop that iterates through each character of
str2
. It starts by pointingp2
to the start ofstr2
. - It compares the character pointed by
p1
with the character pointed byp2
. If they match, it means a matching character is found. - If a match is found, the function returns a pointer to the matching character in
str1
, which is(char *)p1
. - If no match is found, the inner loop continues by incrementing
p2
to the next character instr2
. If there are more characters instr2
, the inner loop continues to the next iteration, comparing the character pointed byp1
with the next character instr2
. - If the inner loop finishes without finding a match, the outer loop continues by incrementing
p1
to the next character instr1
. It then repeats the inner loop to search for a matching character with the next character instr1
. - If the outer loop finishes without finding a match, it means no matching characters were found in
str1
. In this case, the function returnsNULL
. - The calling function can check the return value of
strpbrk()
to determine if any matching characters were found. If the return value isNULL
, it means no matches were found. Otherwise, it points to the first matching character instr1
.
Note: The function stops as soon as it finds a matching character and returns the pointer to that character. It does not necessarily return the first occurrence of the first character in str2
Input / Output
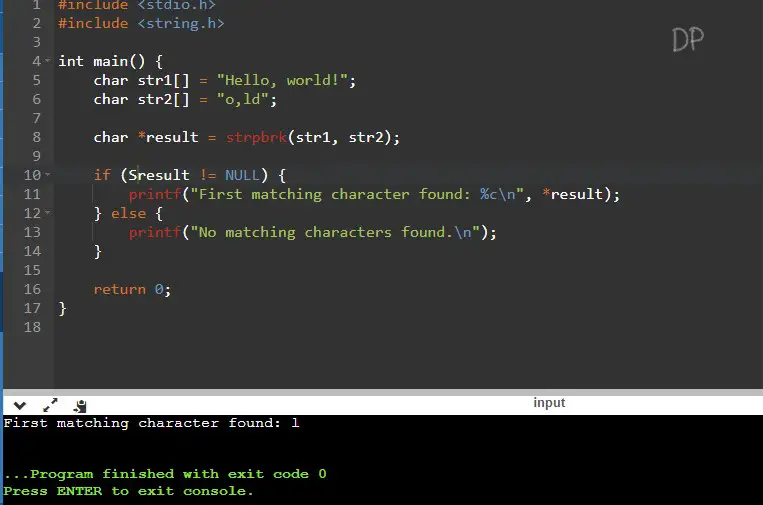