In this C program, we will calculate the volume and surface area of a cube. A cube is a three-dimensional shape with all sides equal in length. The volume of a cube is the measure of space it occupies, while the surface area is the total area of all its faces. By using a few simple mathematical formulas, we can easily compute these values.
Problem statement
Write a C program to calculate the volume and surface area of a cube. The program should prompt the user to enter the length of the cube’s side, and then display the computed volume and surface area.
C Program to Find Volume and Surface Area of a Cube
#include <stdio.h> int main() { float side, volume, surfaceArea; printf("Enter the length of the cube's side: "); scanf("%f", &side); volume = side * side * side; surfaceArea = 6 * side * side; printf("Volume of the cube: %.2f\n", volume); printf("Surface area of the cube: %.2f\n", surfaceArea); return 0; }
Input\Output
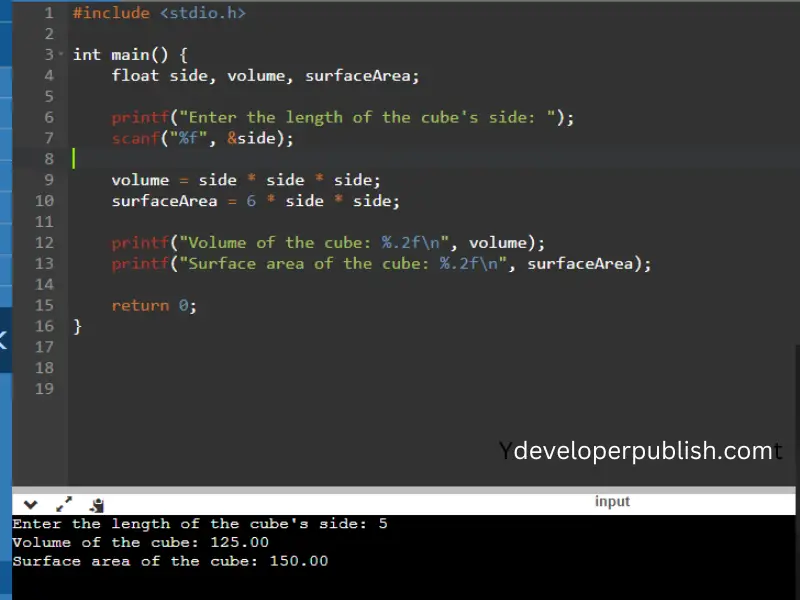