In this C programming tutorial, we will write a program to find the sum of even and odd numbers from 1 to n, where n is a positive integer entered by the user. This program demonstrates the use of loops, conditional statements, and arithmetic operations in C programming.
Problem Statement:
Write a C program to find the sum of even and odd numbers from 1 to n, where n is a positive integer entered by the user.
Solution:
To solve this problem, we will use a for
loop to iterate over the numbers from 1 to n. Inside the loop, we will use an if
statement to check whether the current number is even or odd, and we will update the sum of even and odd numbers accordingly. Here’s the code for our solution:
#include <stdio.h> int main() { int n, i, even_sum = 0, odd_sum = 0; printf("Enter a positive integer: "); scanf("%d", &n); for(i = 1; i <= n; i++) { if(i % 2 == 0) { even_sum += i; } else { odd_sum += i; } } printf("Sum of even numbers from 1 to %d is %d\n", n, even_sum); printf("Sum of odd numbers from 1 to %d is %d\n", n, odd_sum); return 0; }
Output
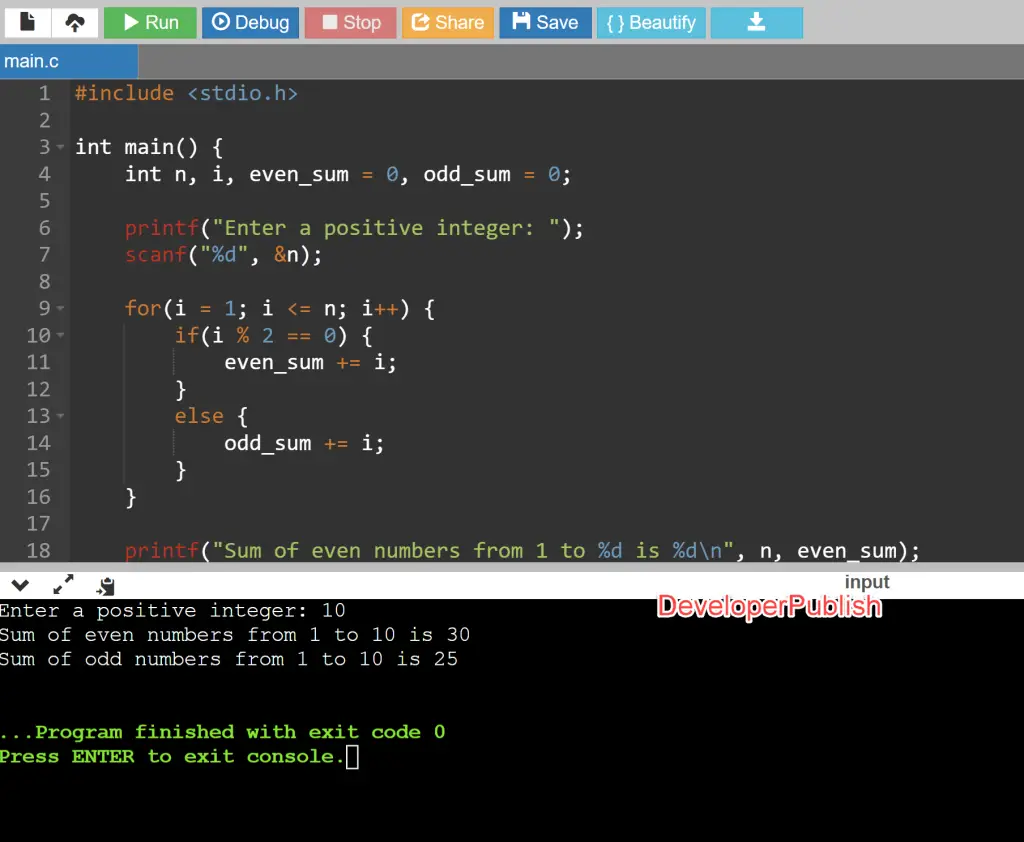
- The
stdio.h
header file is included for standard input/output functions. - The
main()
function is declared as the entry point of the program. - The
start
,end
,even_sum
, andodd_sum
variables are declared.even_sum
andodd_sum
are initialized to zero. - The user is prompted to enter the starting number using
printf()
function. - The user input is read and stored in the
start
variable usingscanf()
function. - The user is prompted to enter the ending number using
printf()
function. - The user input is read and stored in the
end
variable usingscanf()
function. - A
for
loop is used to iterate through the numbers in the range fromstart
toend
. - An
if-else
statement is used to determine whether each number is even or odd. - If the number is even (i.e., the remainder when dividing by 2 is 0), the
even_sum
variable is updated to include the number. - If the number is odd (i.e., the remainder when dividing by 2 is 1), the
odd_sum
variable is updated to include the number. - Once the loop is complete, two
printf()
statements are called to display the sum of even and odd numbers in the given range. - The
main()
function returns 0, which indicates that the program has executed successfully.
Explanation:
The program begins by including the stdio.h
header file, which provides access to standard input/output functions. The main()
function is then declared as the entry point of the program, and the start
, end
, even_sum
, and odd_sum
variables are declared.
Next, the user is prompted to enter the starting number and the ending number using the printf()
function. The scanf()
function is then used to read the user input and store it in the start