This C program calculates and displays the position of the highest bit set (most significant bit) for a given integer. The highest bit set is the leftmost (most significant) bit that is turned on (1) in the binary representation of the integer.
In computer systems, integers are typically represented using a fixed number of bits. Each bit can be either 0 or 1, and the position of a bit determines its value in the integer’s binary representation. The rightmost bit, also known as the least significant bit (LSB), represents the value 2^0 (1), the next bit represents 2^1 (2), the next bit represents 2^2 (4), and so on.
Program Statement
Write a C program that prompts the user to enter an integer and finds the position of the highest bit set in that integer. The program should then display the position of the highest bit set on the console.
- Prompt the user to enter an integer.
- Read the input integer from the user.
- Implement a function,
highestBitSet
, that takes the input integer as a parameter and returns the position of the highest bit set. - Inside the
highestBitSet
function:- Initialize a variable,
bitPos
, to -1 to store the position of the highest bit set. - Use a loop to iterate until the input integer becomes 0.
- In each iteration:
- Right-shift the input integer by 1 bit using the right shift operator (
>>
). - Increment the
bitPos
variable by 1. - If the input integer becomes 0, break out of the loop.
- Right-shift the input integer by 1 bit using the right shift operator (
- Return the
bitPos
value.
- Initialize a variable,
- In the
main
function:- Call the
highestBitSet
function, passing the input integer as an argument. - Receive the result in a variable,
position
. - Display the original input integer and the position of the highest bit set on the console.
- Call the
- End the program.
Ensure that your program handles input validation and accounts for different platforms and representations of integers.
C Program to Find the Highest Bit Set for any Given Integer
#include <stdio.h> int highestBitSet(int num) { int position = 0; // Check each bit starting from the most significant bit for (int i = sizeof(int) * 8 - 1; i >= 0; i--) { // Shift the bit to the rightmost position and check if it is set if ((num >> i) & 1) { position = i; break; } } return position; } int main() { int num; printf("Enter an integer: "); scanf("%d", &num); int highestBit = highestBitSet(num); printf("Position of the highest set bit: %d\n", highestBit); return 0; }
How it Works
- The program prompts the user to enter an integer.
- The
scanf
function reads the input integer from the user and stores it in the variablenum
. - The program calls the
highestBitSet
function and passes the input integernum
as an argument. - Inside the
highestBitSet
function:- The variable
bitPos
is initialized to -1. This variable will store the position of the highest bit set. - The while loop is executed as long as the input integer
num
is greater than 0. - In each iteration of the loop:
- The
num
is right-shifted by 1 bit using the right shift operator (>>
). This moves all the bits one position to the right. - The
bitPos
variable is incremented by 1 to keep track of the current position. - If the input integer
num
becomes 0, the loop is broken.
- The
- Finally, the function returns the value of
bitPos
.
- The variable
- Back in the
main
function:- The returned value from the
highestBitSet
function is stored in the variableposition
. - The program then prints the original input integer
num
and the position of the highest bit set (position
) on the console.
- The returned value from the
By using the right shift operation, the program systematically moves through each bit of the input integer, starting from the most significant bit. The loop continues until the input integer becomes 0, effectively finding the position of the highest bit set. The program then displays the result on the console.
Input/Output
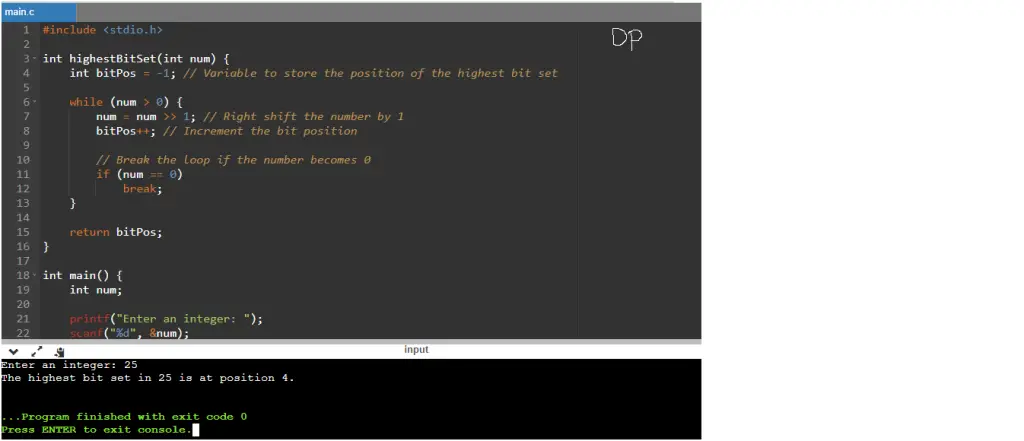