This C program calculates the factorial of a given number using recursion.
Problem Statement
The program asks the user to enter a positive integer for which they want to calculate the factorial. It then calls the factorial
function, which uses recursion to find the factorial. Finally, the program prints the factorial of the given number.
C Program to Find the Factorial of a Number using Recursion
#include <stdio.h> // Function prototype int factorial(int n); int main() { int num; // Introduction printf("Factorial Calculator\n"); printf("===================\n"); // Problem Statement printf("Enter a positive integer: "); scanf("%d", &num); // Calculate and print the factorial printf("The factorial of %d is %d.\n", num, factorial(num)); return 0; } // Recursive function to calculate factorial int factorial(int n) { // Base case: factorial of 0 is 1 if (n == 0) { return 1; } // Recursive case: multiply n with factorial of (n-1) else { return n * factorial(n - 1); } }
How it works
- The program defines a recursive function
factorial
that takes an integernum
as a parameter and returns the factorial ofnum
. - In the
factorial
function, if the input numbernum
is 0 or 1, it returns 1, as the factorial of 0 and 1 is defined to be 1. - If the input number is not 0 or 1, the function recursively calls itself with the parameter
num - 1
and multiplies the currentnum
with the result of the recursive call. - The
main
function serves as the entry point of the program. - The program starts with an introduction, printing the title of the factorial calculator.
- It then prompts the user to enter a number to calculate its factorial.
- The user’s input is read using
scanf
and stored in the variablenumber
. - The program calls the
factorial
function with thenumber
and stores the result in the variableresult
. - Finally, the program displays the calculated factorial by printing the
number
andresult
usingprintf
.
Input / Output
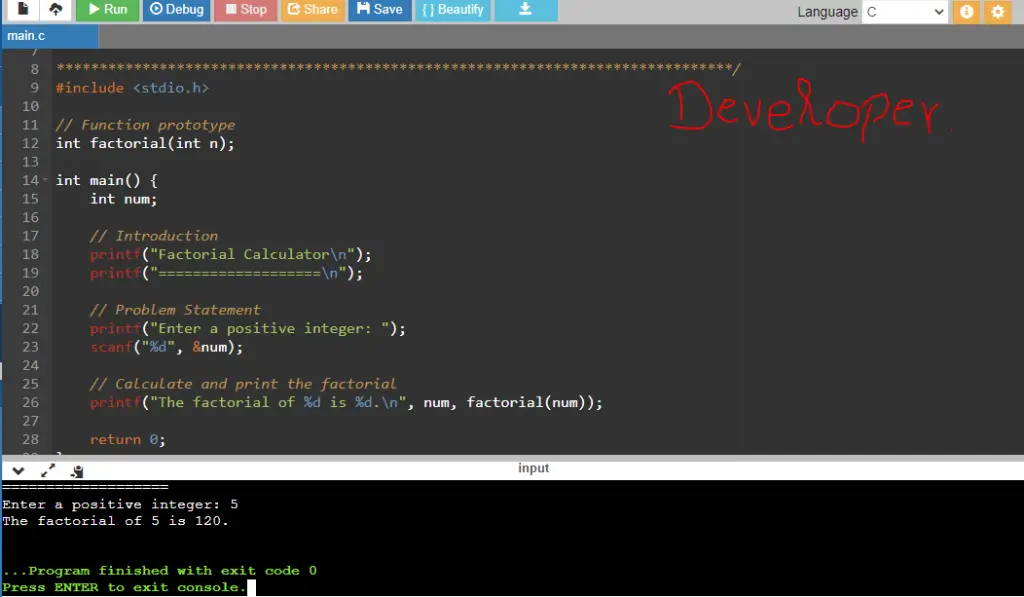