This C program calculates the power of a given number raised to a specified exponent using a loop.
Problem Statement
Given a base number base
and an exponent exponent
, we need to calculate the result of raising the base
to the power of exponent
. The program should use a loop to perform the calculation and display the result.
C Program to Find Power of a Number
#include <stdio.h> int power(int base, int exponent); int main() { int base, exponent, result; // Introduction printf("Power Calculation Program\n"); // Problem Statement printf("Enter the base: "); scanf("%d", &base); printf("Enter the exponent: "); scanf("%d", &exponent); // Program result = power(base, exponent); // Output printf("%d raised to the power of %d is %d\n", base, exponent, result); return 0; } int power(int base, int exponent) { int result = 1; // Calculate power while (exponent > 0) { result *= base; exponent--; } return result; }
How it works
- The program starts with an introduction to the Power Calculation Program.
- It then prompts the user to enter the base and exponent.
- The
power
function is defined, which takes two integers as arguments:base
andexponent
. - Inside the
power
function, a variableresult
is initialized to 1. - The function uses a
while
loop to calculate the power by multiplying thebase
with itselfexponent
number of times. - After the
power
function is called and the result is obtained, it is displayed as the output.
Input / Output
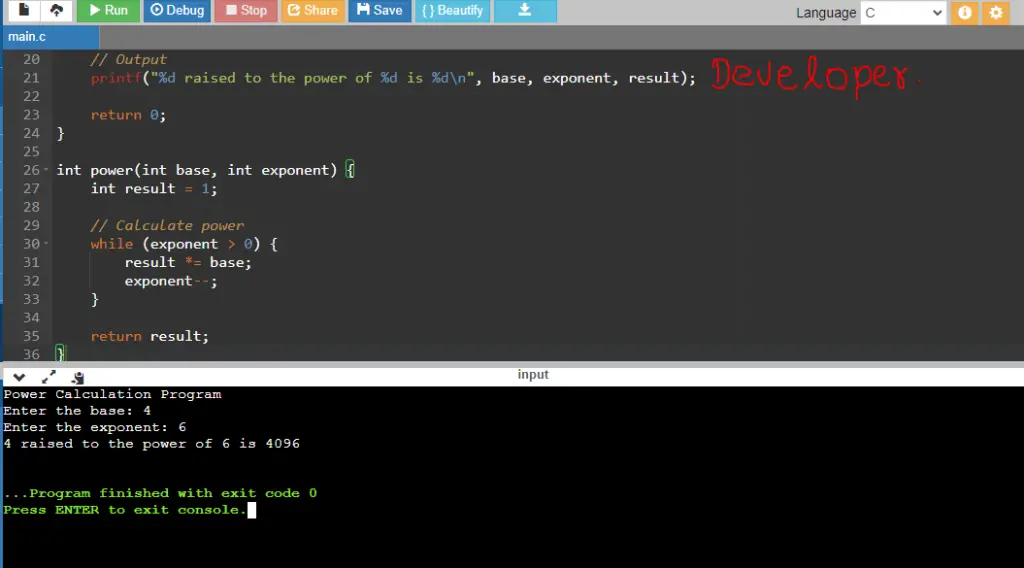