This C program calculates the area of a parallelogram using the base and height provided by the user.
Problem statement
Given the base and height of a parallelogram, we need to calculate its area using the formula area = base * height
. The program should prompt the user to enter the base and height and then display the calculated area on the console.
C Program to Find Area of Parallelogram
#include <stdio.h> int main() { float base, height, area; // Introduction printf("**** Area of Parallelogram Calculator ****\n"); // Problem statement printf("Enter the base length and height of the parallelogram:\n"); // User input scanf("%f %f", &base, &height); // Calculating the area area = base * height; // Output printf("The area of the parallelogram is: %.2f\n", area); return 0; }
How it works
- The program starts with an introduction to indicate the purpose of the program.
- It then prompts the user to enter the base length and height of the parallelogram.
- The program reads the user input using
scanf
and stores the values in the variablesbase
andheight
. - The area of the parallelogram is calculated by multiplying the base length with the height and storing the result in the variable
area
. - Finally, the program displays the calculated area using
printf
.
Input / output
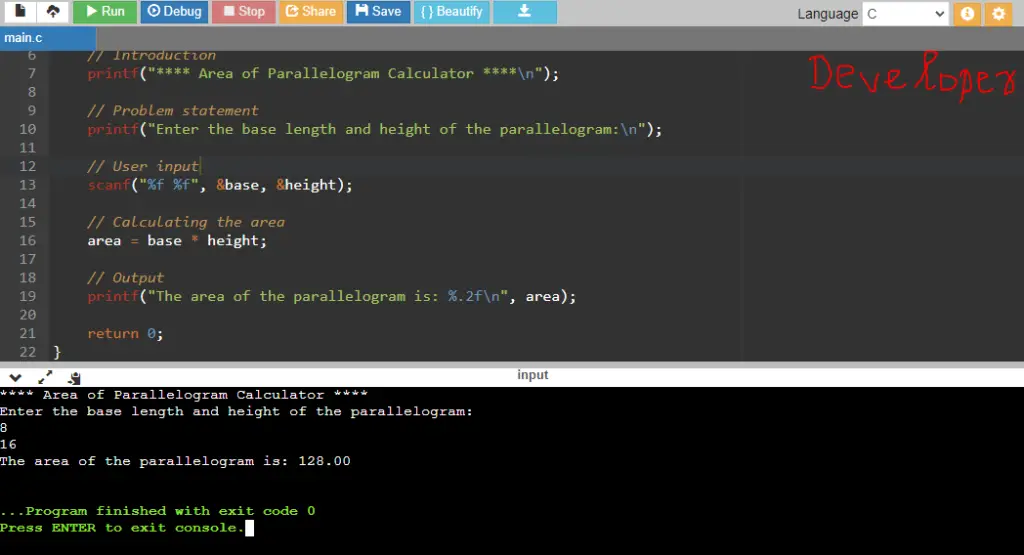