This C program converts decimal numbers to Roman numerals using a function called convertToRoman
. It takes user input, checks if the input is within the valid range, and then calls the conversion function to display the Roman numeral equivalent.
Problem statement
Write a C program that accepts a decimal number between 1 and 3999 from the user and converts it into Roman numerals.
C Program to Convert Numbers to Roman Numerals
// C program to convert numbers to Roman numerals #include <stdio.h> // Function to convert decimal to Roman numerals void convertToRoman(int num) { // Arrays storing Roman numerals and their corresponding values int decimal[] = {1000, 900, 500, 400, 100, 90, 50, 40, 10, 9, 5, 4, 1}; char* roman[] = {"M", "CM", "D", "CD", "C", "XC", "L", "XL", "X", "IX", "V", "IV", "I"}; // Calculate the Roman numeral equivalent int i = 0; while (num > 0) { int quotient = num / decimal[i]; num %= decimal[i]; // Append the Roman numeral equivalent to the output for (int j = 0; j < quotient; j++) { printf("%s", roman[i]); } i++; } } int main() { int number; // Prompt the user for input printf("Enter a number: "); scanf("%d", &number); // Check if the number is within the valid range if (number < 1 || number > 3999) { printf("Invalid input. Please enter a number between 1 and 3999.\n"); return 0; } // Convert the number to Roman numerals and display the result printf("Roman numeral equivalent: "); convertToRoman(number); printf("\n"); return 0; }
How it works?
- The program begins by defining a function called
convertToRoman
that takes an integernum
as a parameter. - Inside the function, two arrays are declared:
decimal[]
to store the decimal values of Roman numerals androman[]
to store the corresponding Roman numeral characters. - The function uses a while loop to iterate through the decimal values, starting with the largest (1000) and moving to the smallest (1).
- In each iteration, the function calculates the quotient of
num
divided by the current decimal value and stores it in the variablequotient
. It then calculates the remainder of the division and updatesnum
accordingly. - A nested for loop is used to print the Roman numeral character
quotient
number of times. - After the loop finishes, the function returns to the main program.
- In the
main
function, the user is prompted to enter a number. The input is read usingscanf
and stored in the variablenumber
. - The program checks if the entered number is within the valid range (1 to 3999) and displays an error message if it’s not.
- If the number is valid, the program calls the
convertToRoman
function and passes thenumber
as an argument. - The Roman numeral equivalent is printed on the screen.
Input/Output
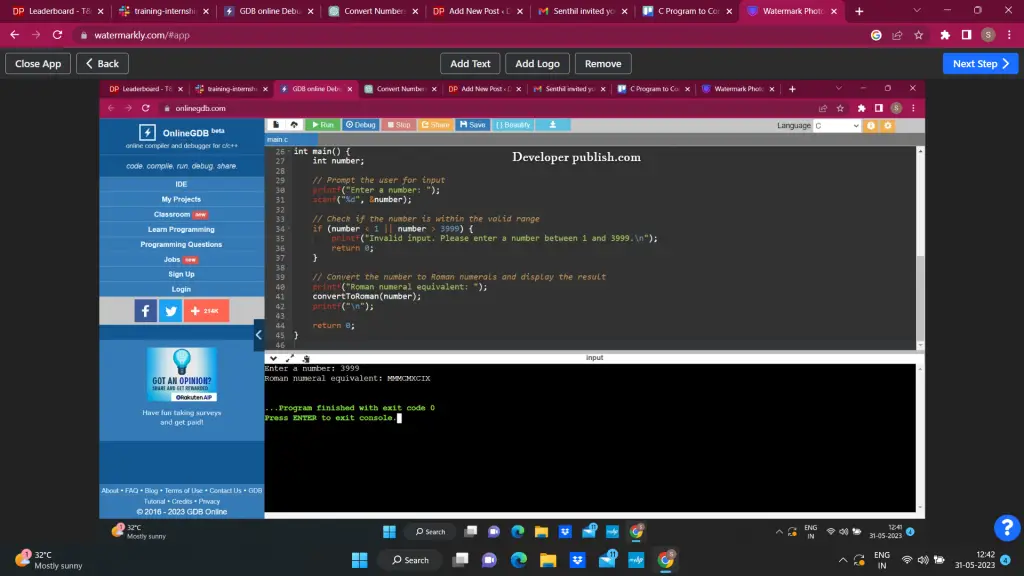