This C program compares two strings entered by the user and determines their relationship based on lexicographical order.
Problem statement
Given two strings, we need to compare them and determine their relationship in lexicographical order. The program should indicate if the strings are equal or which one comes first in dictionary order.
C program to Compare Two Strings
#include <stdio.h> #include <string.h> int main() { char str1[100], str2[100]; int result; // Introduction printf("String Comparison Program\n"); // Problem statement printf("Enter the first string: "); gets(str1); printf("Enter the second string: "); gets(str2); // Compare strings result = strcmp(str1, str2); // How it works if (result == 0) { printf("Both strings are equal.\n"); } else if (result < 0) { printf("String 1 is lexicographically smaller than String 2.\n"); } else { printf("String 1 is lexicographically greater than String 2.\n"); } // Input/Output printf("\n--- INPUT ---\n"); printf("String 1: %s\n", str1); printf("String 2: %s\n", str2); printf("\n--- OUTPUT ---\n"); printf("Comparison Result: %d\n", result); return 0; }
How it works
In this program, we compare two strings using the strcmp()
function from the string.h
library. The strcmp()
function returns an integer value that indicates the lexicographical relationship between the two strings. If the return value is 0, it means the strings are equal. A negative value indicates that the first string is lexicographically smaller, and a positive value indicates that the first string is lexicographically greater.
Input / output
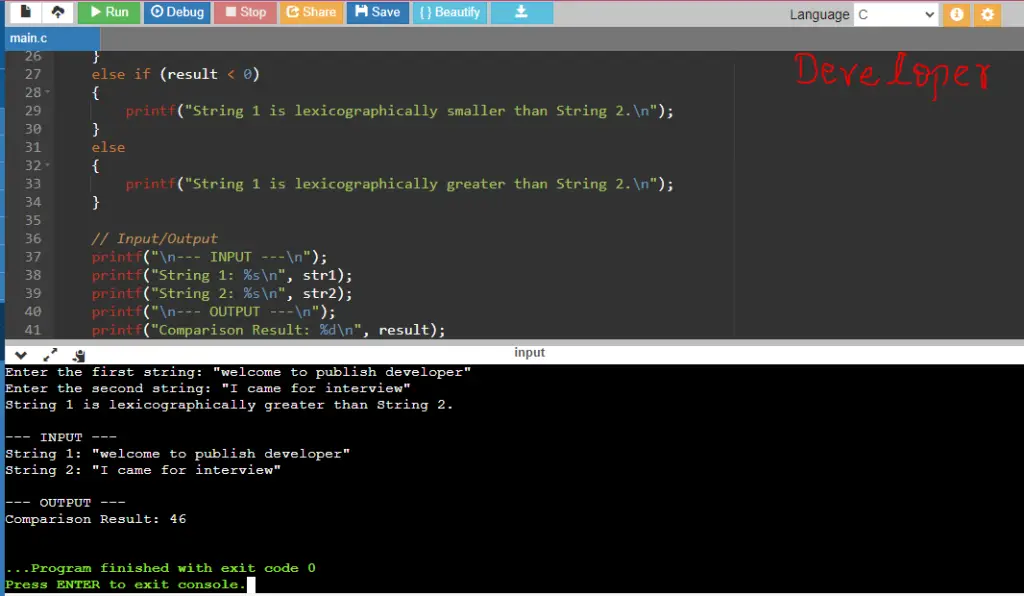