This C program first introduces itself as a “Fahrenheit to Celsius Converter”. It then provides a brief problem statement, explaining that it converts temperature from Fahrenheit to Celsius.
Problem Statement
In the program, we declare two variables fahrenheit
and celsius
of type float
. The user is prompted to enter the temperature in Fahrenheit. The program reads the input using scanf
and stores it in the fahrenheit
variable.
The formula to convert Fahrenheit to Celsius is (F - 32) * 5/9
. We calculate the value of celsius
using this formula.
C program to Convert Fahrenheit to Celsius
#include <stdio.h> int main() { // Intro printf("Fahrenheit to Celsius Converter\n\n"); // Problem Statement printf("This program converts temperature from Fahrenheit to Celsius.\n\n"); // Program float fahrenheit, celsius; // How it works printf("Enter temperature in Fahrenheit: "); scanf("%f", &fahrenheit); celsius = (fahrenheit - 32) * 5 / 9; // Input / Output printf("\n%.2f degrees Fahrenheit is equal to %.2f degrees Celsius.\n", fahrenheit, celsius); return 0; }
How it works
The program then prompts the user to enter the temperature in Fahrenheit and reads the input using scanf
. The entered value is stored in the fahrenheit
variable.
Next, the program performs the conversion using the formula (F - 32) * 5/9
, where F
represents the temperature in Fahrenheit. The result is stored in the celsius
variable.
Finally, the converted temperature in Celsius is displayed on the screen using printf
, with the input and output values displayed with 2 decimal places.
Input / Output
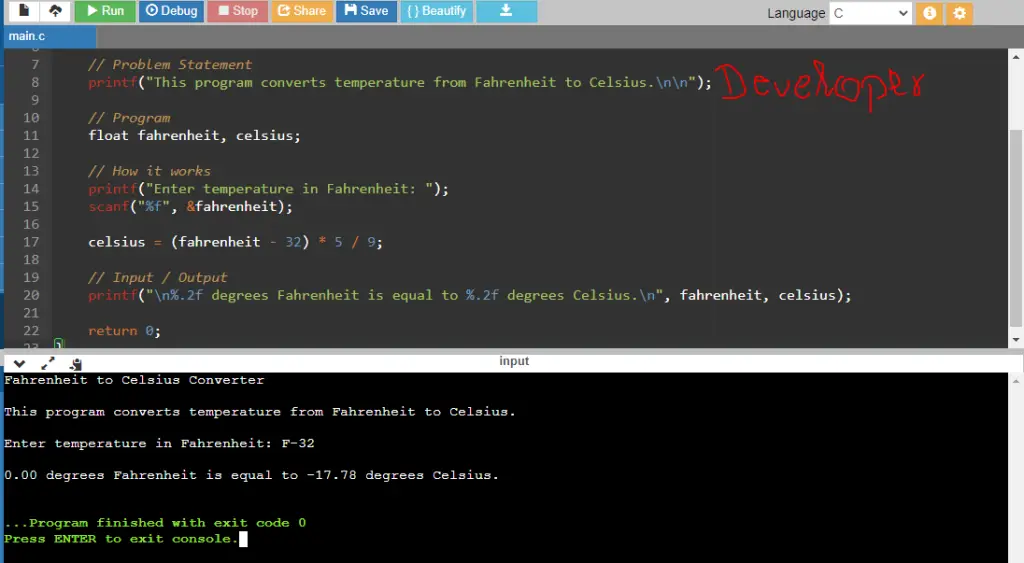