This C program converts a decimal number into its hexadecimal representation.
Hexadecimal (or hex) is a base-16 numbering system that uses 16 unique digits to represent values from 0 to 15. The digits 0-9 represent their decimal counterparts, and the letters A-F (or a-f) represent the values 10-15.
Problem Statement
Write a C program that converts a decimal number to its hexadecimal representation.
The program should perform the following steps:
- Prompt the user to enter a decimal number.
- Read the decimal number input from the user.
- Implement a function,
decimalToHexadecimal
, which takes the decimal number as input and converts it to its hexadecimal equivalent. - Within the
decimalToHexadecimal
function, perform the conversion by repeatedly dividing the decimal number by 16 and obtaining the remainder. Map each remainder to its corresponding hexadecimal digit. - Store the hexadecimal digits in an array.
- Print the resulting hexadecimal representation by iterating through the array in reverse order.
- Finally, in the
main
function, call thedecimalToHexadecimal
function with the user-provided decimal number and display the hexadecimal representation.
Note:
- The program should handle decimal numbers within the range of integers supported by the C language.
- The program should handle the conversion for positive decimal numbers only.
You can use the provided C program template in the previous response as a starting point to solve the problem.
C program to Convert Decimal to Hexadecimal
#include <stdio.h> void decimalToHexadecimal(int decimalNumber) { int quotient, remainder; char hexadecimalNumber[100]; int i = 0; quotient = decimalNumber; while (quotient != 0) { remainder = quotient % 16; if (remainder < 10) hexadecimalNumber[i++] = remainder + 48; else hexadecimalNumber[i++] = remainder + 55; quotient = quotient / 16; } printf("Hexadecimal representation: "); for (int j = i - 1; j >= 0; j--) printf("%c", hexadecimalNumber[j]); printf("\n"); } int main() { int decimalNumber; printf("Enter a decimal number: "); scanf("%d", &decimalNumber); decimalToHexadecimal(decimalNumber); return 0; }
How it Works
- The program begins by including the necessary header file,
<stdio.h>
, to enable input and output operations. - The
decimalToHexadecimal
function is defined to convert the decimal number to its hexadecimal representation. It takes an integer parameter,decimalNumber
, which is the decimal value to be converted. - Inside the
decimalToHexadecimal
function:- Variables are declared:
quotient
to store the quotient obtained during the division process.remainder
to store the remainder obtained during each division.hexadecimalNumber
as an array of characters to store the hexadecimal digits of the converted number.i
as an index variable to keep track of the position of each digit in thehexadecimalNumber
array.
- The
quotient
is initially set to thedecimalNumber
. - A loop is used to perform the conversion process:
- In each iteration, the
remainder
is calculated as the remainder when thequotient
is divided by 16. This step is performed using the modulus operator%
. - If the
remainder
is less than 10, it represents a digit between 0 and 9. In this case, the ASCII value of the digit is calculated by adding 48 to theremainder
. The resulting character is stored in thehexadecimalNumber
array at indexi
. - If the
remainder
is greater than or equal to 10, it represents a digit between A and F. In this case, the ASCII value of the digit is calculated by adding 55 to theremainder
(to account for the ASCII values of A, B, C, D, E, and F). The resulting character is stored in thehexadecimalNumber
array at indexi
. - The
quotient
is updated by dividing it by 16 to move on to the next iteration. - The loop continues until the
quotient
becomes 0, indicating that the conversion is complete.
- In each iteration, the
- After the loop, the resulting hexadecimal representation is printed by iterating through the
hexadecimalNumber
array in reverse order. This is done by starting the loop fromi-1
and decrementingj
until it becomes 0. The hexadecimal digits are printed as characters using theprintf
function.
- Variables are declared:
- In the
main
function:- An integer variable
decimalNumber
is declared to store the decimal number entered by the user. - The user is prompted to enter a decimal number using the
printf
function. - The decimal number is read from the user using the
scanf
function and stored in thedecimalNumber
variable. - The
decimalToHexadecimal
function is called with thedecimalNumber
as an argument to perform the conversion and display the hexadecimal representation.
- An integer variable
By following these steps, the program takes a decimal number as input, converts it to its hexadecimal representation using the decimalToHexadecimal
function, and displays the result to the user.
Input /Output
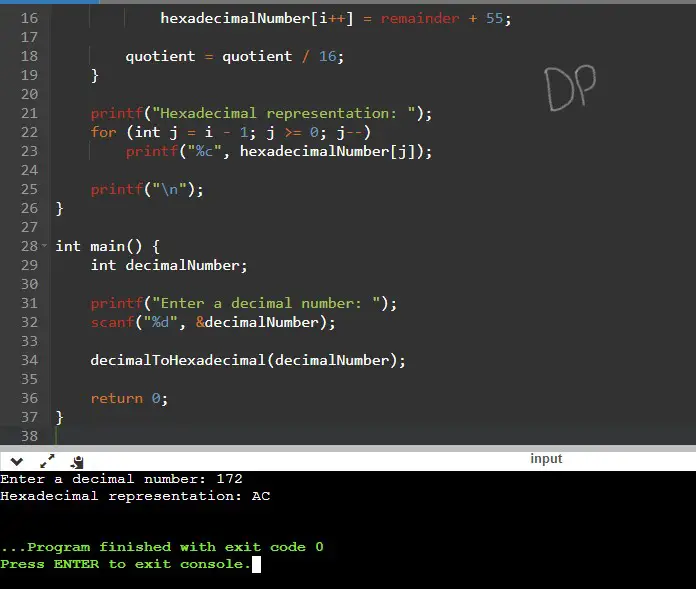
In this example, the program prompts the user to enter a decimal number. The user enters the number 172
.
The program then converts the decimal number 172
to its hexadecimal representation using the decimalToHexadecimal
function. The resulting hexadecimal representation is AC
.
Finally, the program displays the hexadecimal representation to the user as AC
.
You can run the program and try different decimal numbers to see their corresponding hexadecimal representations.