This program provides a basic structure for converting days into years, months, and days in C#. You can run this program by compiling and executing it using a C# compiler.
Problem statement
You are tasked with creating a C# program that converts a given number of days into years, months, and remaining days. Keep in mind that this program assumes a year has 365 days and a month has 30 days for simplicity.
C# Program to Convert Days into Years, Months and Days
using System; class Program { static void Main() { Console.Write("Enter the number of days: "); int days = Convert.ToInt32(Console.ReadLine()); int years = days / 365; int remainingDays = days % 365; int months = remainingDays / 30; int finalDays = remainingDays % 30; Console.WriteLine($"{days} days is approximately:"); Console.WriteLine($"{years} years, {months} months, and {finalDays} days."); } }
How it works
The C# program provided converts a given number of days into years, months, and remaining days. Here’s a step-by-step explanation of how it works:
- User Input:
- The program starts by prompting the user to enter the number of days using
Console.Write("Enter the number of days: ");
.
- The program starts by prompting the user to enter the number of days using
- Reading User Input:
- The user’s input is read as a string using
Console.ReadLine()
. - The
Convert.ToInt32()
method is used to convert the input from a string to an integer.
- The user’s input is read as a string using
- Calculating Years, Months, and Days:
- The program uses integer division and modulus operations to convert the total number of days into years, months, and remaining days.
int years = days / 365;
calculates the number of whole years.int remainingDays = days % 365;
computes the remaining days after subtracting the years.int months = remainingDays / 30;
determines the number of whole months.int finalDays = remainingDays % 30;
computes the remaining days after subtracting the months.
- Displaying the Result:
- The program then uses
Console.WriteLine()
to display the converted values in a user-friendly format. - For example,
Console.WriteLine($"{years} years, {months} months, and {finalDays} days.");
prints the result.
- The program then uses
- Execution:
- When the user runs the program, it will prompt for input, receive the number of days, and perform the necessary calculations.
- Example Output:
- If the user enters 1500 days, the program will output:
Code:
1500 days is approximately:
4 years, 1 month, and 20 days.
This program provides a basic conversion of days into years, months, and remaining days. Keep in mind that this is a simplified calculation and assumes a year has 365 days and a month has 30 days for ease of computation. In real-world scenarios, the number of days in a month varies.
Input/Output
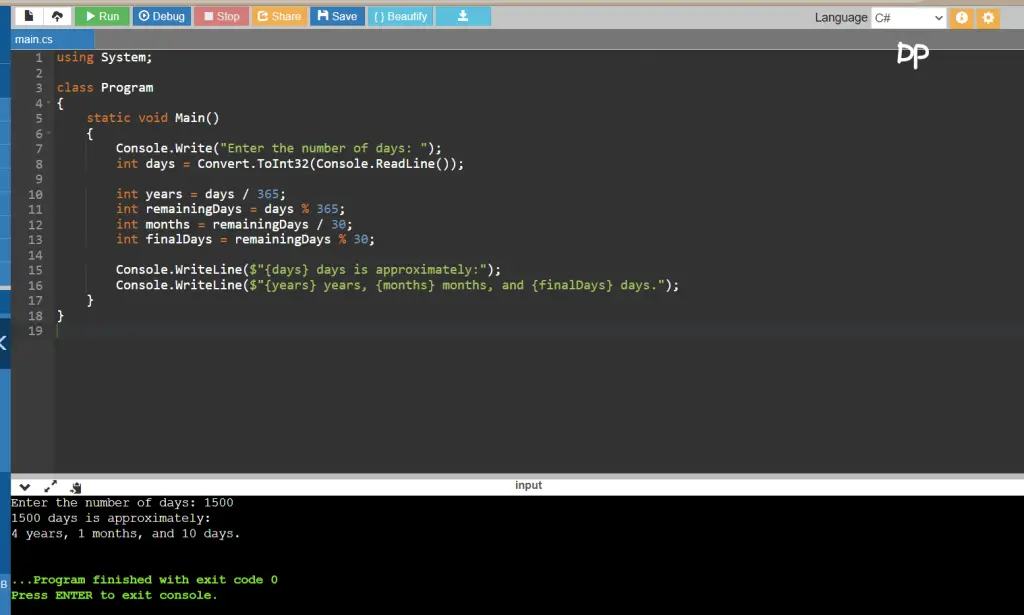