This C program converts a given number of days into years, months, and remaining days. It uses a function called convertDays
to perform the conversion.
Problem statement
Given a number of days, the program needs to determine the equivalent number of years, months, and remaining days.
C Program to Convert Days into Years, Months and Days
#include <stdio.h> void convertDays(int days, int *years, int *months, int *remainingDays) { *years = days / 365; *months = (days % 365) / 30; *remainingDays = (days % 365) % 30; } int main() { int days, years, months, remainingDays; // Input printf("Enter the number of days: "); scanf("%d", &days); // Conversion convertDays(days, &years, &months, &remainingDays); // Output printf("Years: %d\n", years); printf("Months: %d\n", months); printf("Days: %d\n", remainingDays); return 0; }
How it works?
- The user enters the number of days.
- The
convertDays
function is called with the input days and the addresses ofyears
,months
, andremainingDays
. - Inside the
convertDays
function, the number of years is calculated by dividing the days by 365 (approximating a year to 365 days). - The remaining days after considering the years are calculated by taking the modulus of days with 365.
- The number of months is calculated by dividing the remaining days by 30 (approximating a month to 30 days).
- The remaining days after considering the months are calculated by taking the modulus of remaining days with 30.
- The calculated values of years, months, and remaining days are stored in the respective variables.
- The program returns to the
main
function. - The converted values of years, months, and remaining days are printed on the screen.
Input/Output
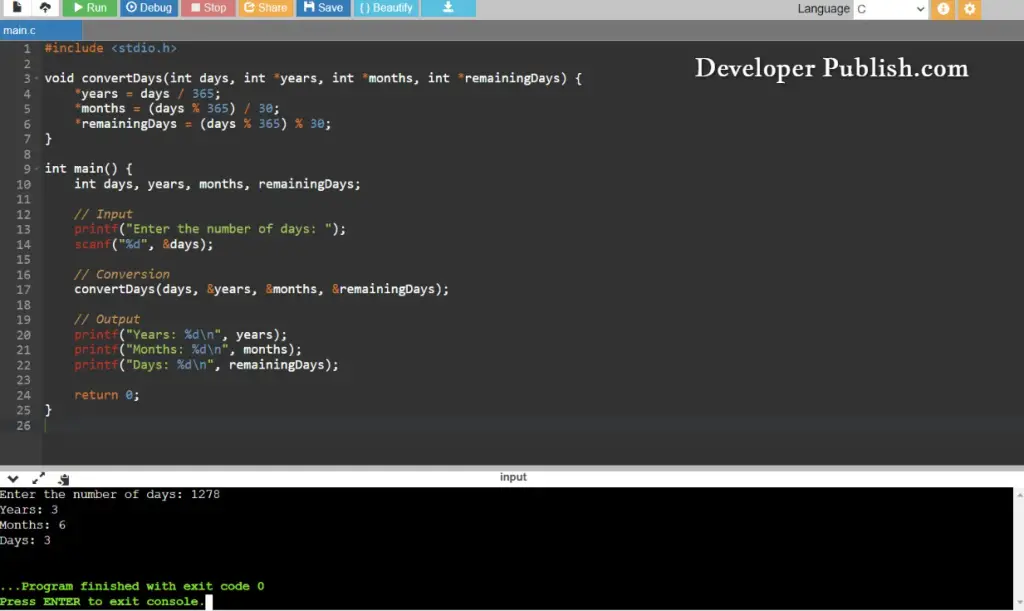