This C# program is designed to convert a positive integer into its negative counterpart. The process is achieved by utilizing a simple mathematical operation – multiplying the positive number by -1. The program provides a clear user interface, guiding the user to input a positive number, processing the conversion, and then displaying both the original positive number and its corresponding negative value.
Problem statement
You are required to write a C# program that converts a positive integer into its negative counterpart. Your program should take a positive integer as input, perform the conversion, and then display the resulting negative number.
C# Program to Convert a Positive Number into Negative
using System; class Program { static void Main() { Console.Write("Enter a positive number: "); int positiveNumber = Convert.ToInt32(Console.ReadLine()); int negativeNumber = ConvertToNegative(positiveNumber); Console.WriteLine($"The negative of {positiveNumber} is {negativeNumber}"); } static int ConvertToNegative(int number) { return -number; } }
How it works
- Program Structure:
- The program starts with the declaration of the
Program
class. - Inside this class, we define two methods:
Main
andConvertToNegative
.
- The program starts with the declaration of the
- Main Method:
- The
Main
method is the entry point of the program. - It first prompts the user to enter a positive number using
Console.Write("Enter a positive number: ")
. - It then reads the user’s input using
Console.ReadLine()
and converts it to an integer usingConvert.ToInt32(...)
. - This positive number is stored in the variable
positiveNumber
.
- The
- ConvertToNegative Method:
- This method takes an integer
number
as input and returns its negation. - Inside the method, it uses the unary minus (
-
) operator to negate the input and returns the result.
- This method takes an integer
- Main Method (Continued):
- After obtaining the positive number, the program calls the
ConvertToNegative
method, passingpositiveNumber
as an argument. This converts the positive number into its negative equivalent. - The result is stored in the variable
negativeNumber
.
- After obtaining the positive number, the program calls the
- Display the Result:
- Finally, the program uses
Console.WriteLine(...)
to display the original positive number and its negative counterpart. It uses string interpolation ($
) to incorporate the variables into the output message.
25
as the positive number, the program will output: - Finally, the program uses
Code: The negative of 25 is -25
- The program works by taking a positive number, passing it through the
ConvertToNegative
method, and then displaying the original and negated values.
Please note that the program assumes that the user will input a valid positive integer. If the user enters something that’s not a valid integer (like a letter or a decimal), the program might throw an exception. In a real-world application, you would want to add error handling to account for such cases.
Input/Output
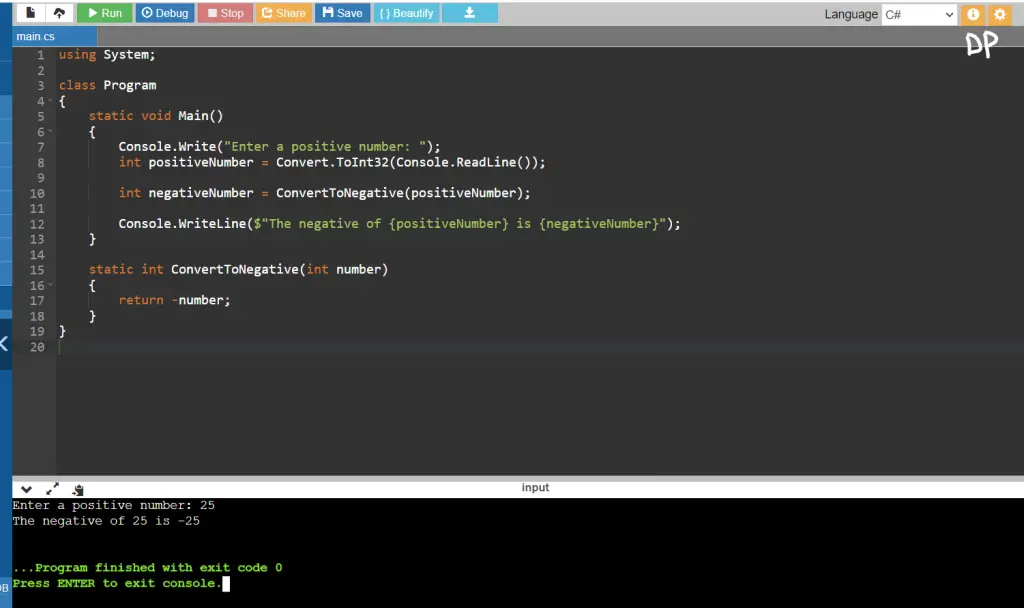