In this C program, we will calculate the power of a number using the exponentiation operator. We will take the base value ‘x’ and the exponent value ‘n’ from the user, and then calculate the result of x raised to the power of n.
Problem statement
Write a C program to calculate the power of a number using the exponentiation operator.
C Program to Calculate Pow (x,n)
#include<stdio.h> #include<math.h> double calculatePower(double x, int n); int main() { double x; int n; double result; printf("Enter the base value (x): "); scanf("%lf", &x); printf("Enter the exponent value (n): "); scanf("%d", &n); result = calculatePower(x, n); printf("Result: %.2lf\n", result); return 0; } double calculatePower(double x, int n) { return pow(x, n); }
How it works
- We include the necessary header files
stdio.h
andmath.h
. Thestdio.h
header file provides input/output functions, and themath.h
header file provides mathematical functions such aspow
. - We declare a function
calculatePower
that takes a double valuex
as the base and an integer valuen
as the exponent. This function uses thepow
function frommath.h
to calculate and return the result of x raised to the power of n. - In the
main
function, we declare variablesx
,n
, andresult
. - We prompt the user to enter the base value (x) and read it using
scanf
with the%lf
format specifier for a double. - We prompt the user to enter the exponent value (n) and read it using
scanf
with the%d
format specifier for an integer. - We call the
calculatePower
function, passingx
andn
as arguments, and assign the returned value to theresult
variable. - Finally, we display the result using
printf
with the%.2lf
format specifier to display the result with two decimal places.
Input / Output
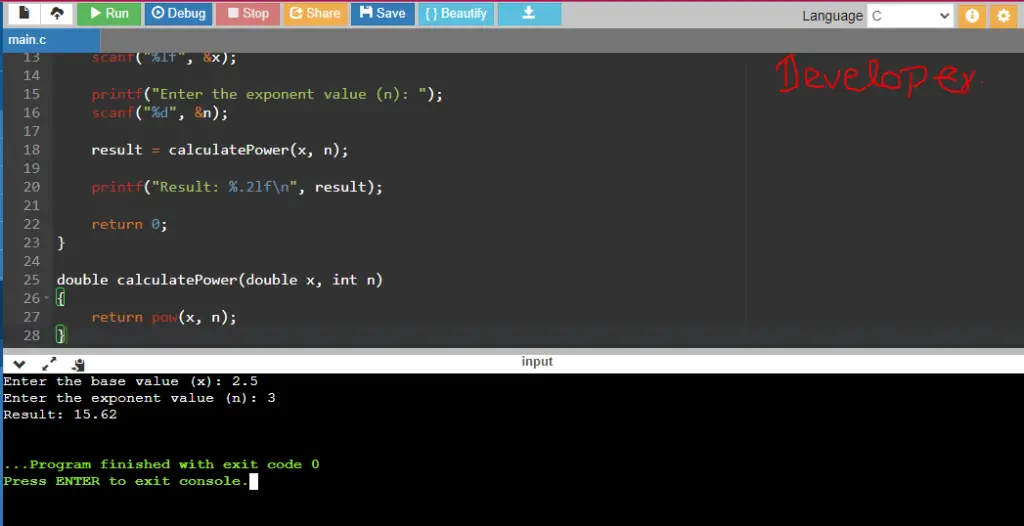