The Acceleration Calculator is a C# program designed to swiftly compute acceleration based on user-provided initial and final velocities, along with the time taken for the change in velocity. Acceleration, a fundamental concept in physics, represents the rate of change of velocity with respect to time.
Problem statement
You are tasked with creating a C# program that calculates acceleration based on user input.
C# Program to Calculate Acceleration
using System; class Program { static void Main() { double initialVelocity, finalVelocity, time; Console.WriteLine("Enter initial velocity (m/s): "); initialVelocity = Convert.ToDouble(Console.ReadLine()); Console.WriteLine("Enter final velocity (m/s): "); finalVelocity = Convert.ToDouble(Console.ReadLine()); Console.WriteLine("Enter time taken (s): "); time = Convert.ToDouble(Console.ReadLine()); double acceleration = CalculateAcceleration(initialVelocity, finalVelocity, time); Console.WriteLine("The acceleration is: " + acceleration + " m/s^2"); } static double CalculateAcceleration(double initialVelocity, double finalVelocity, double time) { return (finalVelocity - initialVelocity) / time; } }
How it works
How the Acceleration Calculator Works:
- User Input:
- The program begins by prompting the user to provide three pieces of information: the initial velocity (initialvinitial), the final velocity (finalvfinal), and the time (t) taken for the change in velocity.
- Data Input:
- The user responds by entering these values using the console interface.
- Calculation:
- Once the user input is received, the program employs the provided formula: a=tvfinal−vinitial to compute the acceleration (a).
- Acceleration Output:
- The program displays the calculated acceleration to the user.
- Program Completion:
- The program execution concludes, providing the user with the desired acceleration value.
- Further Use (Optional):
- If the user wishes to calculate acceleration for different sets of initial and final velocities, they can restart the program and follow the prompts once again.
The program operates with precision, offering an efficient and reliable means to determine acceleration based on the provided inputs. It adheres to the fundamental principles of physics, allowing users to explore and understand the concept of acceleration in a practical context.
Input/Output
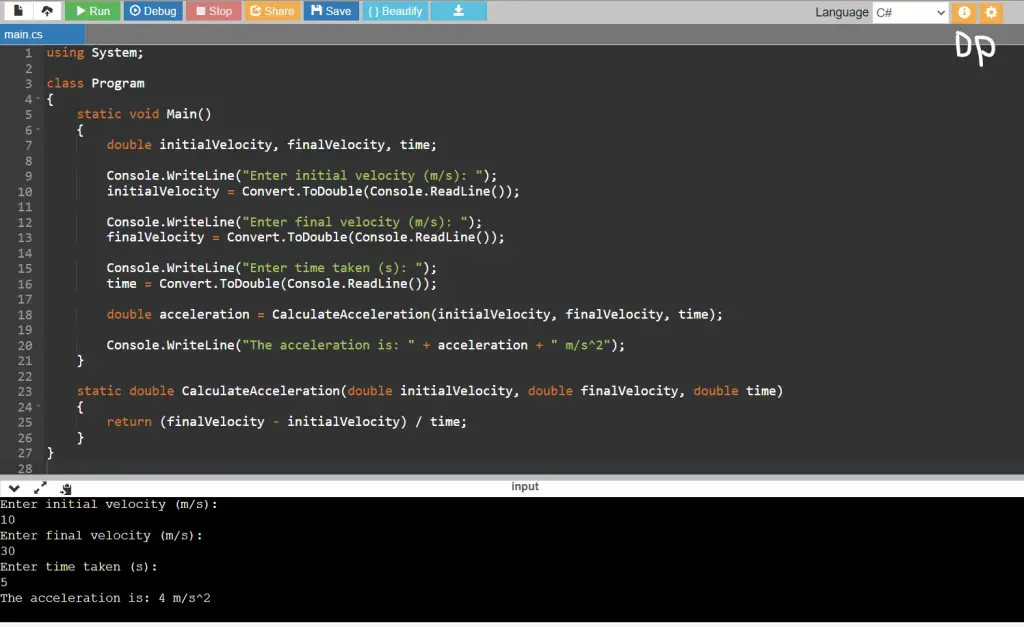