This C# program demonstrates the process of copying a specified section of one array to another array. Arrays in C# are collections of elements, and you might want to extract and duplicate a part of an array into another array for various data manipulation tasks.
Problem Statement
You are given a source array containing integers and an empty destination array. Your task is to implement a function that copies a specified section of the source array to the destination array.
C# Program to Copy a Section of One Array to Another
using System; class Program { static void Main() { int[] sourceArray = { 1, 2, 3, 4, 5 }; int[] destinationArray = new int[3]; // Create a destination array with the desired size int sourceIndex = 1; // Index to start copying from in the source array int destinationIndex = 0; // Index to start copying to in the destination array int length = 3; // Number of elements to copy // Copy the section from sourceArray to destinationArray Array.Copy(sourceArray, sourceIndex, destinationArray, destinationIndex, length); // Display the contents of the destination array Console.WriteLine("Destination Array:"); foreach (int item in destinationArray) { Console.WriteLine(item); } } }
How it Works
- Function Signature: This function takes the following parameters:
sourceArray
: The array containing the source data.sourceIndex
: The index in the source array where copying should start.destinationArray
: The array that will receive the copied elements.destinationIndex
: The index in the destination array where copying should start.length
: The number of elements to copy from the source array to the destination array.
- Copy Elements:Inside the function, you will use a loop to copy the specified section of
sourceArray
todestinationArray
. This involves iterating through the source array starting fromsourceIndex
and copyinglength
elements to the destination array starting fromdestinationIndex
.This loop copies each element one by one, taking care to use the correct indices in both arrays. - Return the Result:After copying the elements, your function should return the modified
destinationArray
, which now contains the copied section of the source array.csharpCopy codereturn destinationArray;
- Example:You can call this function with sample input as shown in the problem statement: After the function call, the
result
array should contain[2, 3, 4]
, which represents the section of the source array that was copied. - Edge Cases:Ensure that your code handles edge cases, such as:
- Checking if the destination array has enough space to accommodate the specified section. You may need to resize the destination array if necessary.
- Validating that the provided indices and length are within the bounds of the source and destination arrays to prevent runtime errors.
Input/ Output
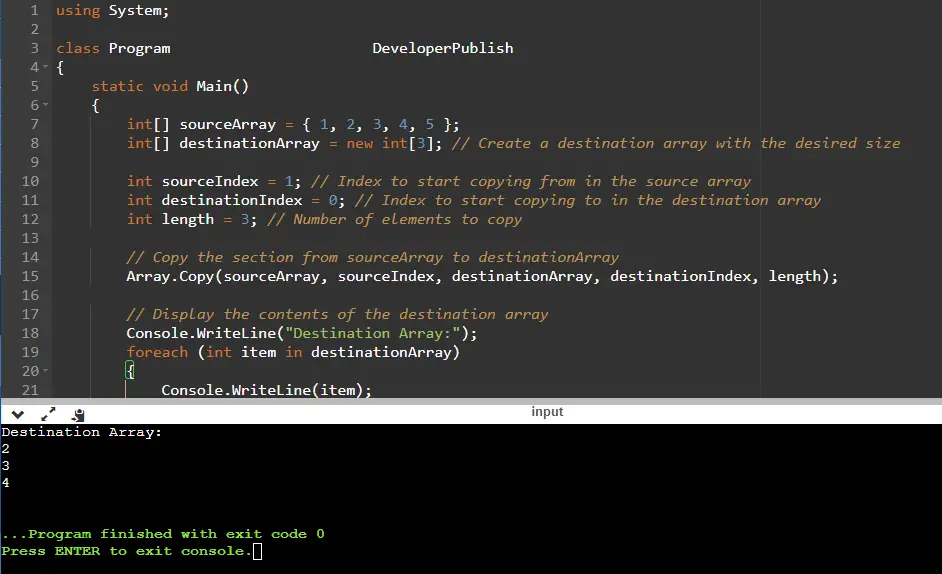