This Python program is designed to analyze a list of numbers and compute the sums of specific categories within that list. It categorizes the numbers into three groups: negative numbers, positive even numbers, and positive odd numbers. Subsequently, it calculates the sum for each category and prints out the results.
Problem Statement
You are tasked with creating a Python program that performs analysis on a list of integers.
Python Program to Print Sum of Negative Numbers, Positive Even & Odd Numbers in a List
def calculate_sums(numbers): sum_negative = 0 sum_even = 0 sum_odd = 0 for num in numbers: if num < 0: sum_negative += num elif num % 2 == 0: sum_even += num else: sum_odd += num return sum_negative, sum_even, sum_odd # Input list of numbers numbers = [12, -7, 5, 64, -14] # Calculate the sums sum_negative, sum_even, sum_odd = calculate_sums(numbers) # Print the results print("Sum of negative numbers:", sum_negative) print("Sum of positive even numbers:", sum_even) print("Sum of positive odd numbers:", sum_odd)
How it works
The Python program provided calculates and prints the sum of negative numbers, positive even numbers, and positive odd numbers in a list. Here’s a step-by-step explanation of how it works:
- Define the
calculate_sums
Function:def calculate_sums(numbers):
- This function is defined to calculate the sums. It takes a list of integers (
numbers
) as input.
- Initialize Sum Variables:
sum_negative = 0 sum_even = 0 sum_odd = 0
- Three variables (
sum_negative
,sum_even
, andsum_odd
) are initialized to keep track of the sums for negative numbers, positive even numbers, and positive odd numbers, respectively. These variables are initially set to 0.
- Iterate Through the List:
for num in numbers:
- The program uses a
for
loop to iterate through each number in the input list.
- Categorize and Sum the Numbers:
if num < 0: sum_negative += num elif num % 2 == 0: sum_even += num else: sum_odd += num
- For each number in the list, it checks whether the number is:
- Negative: If yes, it adds the number to
sum_negative
. - Positive and even: If yes, it adds the number to
sum_even
. - Positive and odd: If yes, it adds the number to
sum_odd
.
- Return the Sums:
return sum_negative, sum_even, sum_odd
- The function returns the calculated sums as a tuple containing
sum_negative
,sum_even
, andsum_odd
.
- Input List and Calculate Sums:
numbers = [12, -7, 5, 64, -14] sum_negative, sum_even, sum_odd = calculate_sums(numbers)
- In the main part of the program, a list of numbers (
numbers
) is defined. Thecalculate_sums
function is then called with this list as input, and the results are assigned tosum_negative
,sum_even
, andsum_odd
.
- Print the Results:
print("Sum of negative numbers:", sum_negative) print("Sum of positive even numbers:", sum_even) print("Sum of positive odd numbers:", sum_odd)
- Finally, the program prints the results with clear labels, indicating the sum of negative numbers, the sum of positive even numbers, and the sum of positive odd numbers.
- By following these steps, the program processes a list of numbers and categorizes them into the specified categories, calculating and printing the sums for each category.
Input/Output
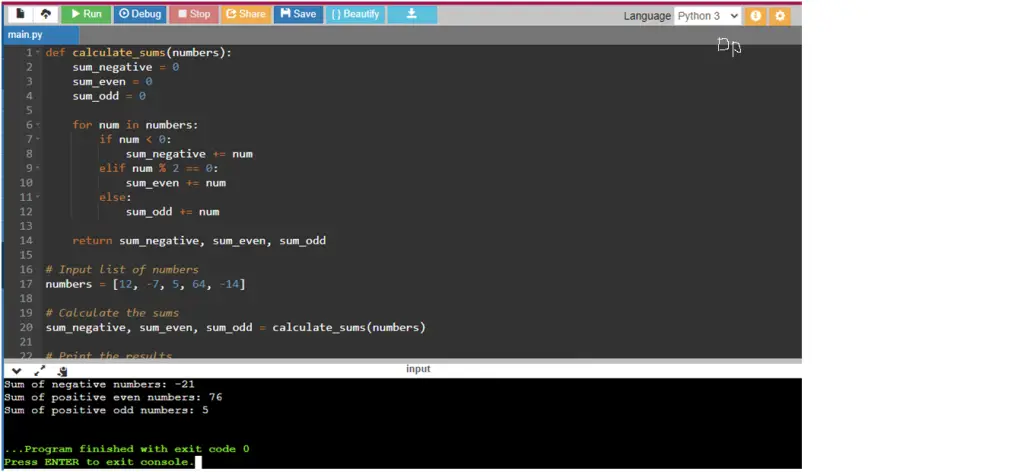