This Python program takes a dictionary as input and multiplies all the values in the dictionary. It then returns the result of the multiplication.
Problem Statement:
You are given a dictionary containing numeric values. Your task is to write a program that calculates the product of all the values in the dictionary and returns the result.
Python Program to Multiply All the Items in a Dictionary
def multiply_dictionary_values(input_dict): result = 1 for value in input_dict.values(): result *= value return result # Input dictionary input_dict = { 'a': 3, 'b': 4, 'c': 2 } # Calculate the product of dictionary values product = multiply_dictionary_values(input_dict) # Output the result print("The product of all dictionary values:", product)
How it Works:
- The program defines a function
multiply_dictionary_values
that takes a dictionaryinput_dict
as an argument. - The variable
result
is initialized to 1, which will be used to store the product of all dictionary values. - The program then iterates through each value in the
input_dict
using a loop. - Inside the loop, each value is multiplied with the current value of
result
. - After iterating through all the values, the final product is stored in the
result
variable and returned. - The input dictionary is provided with sample values.
- The program calculates the product of values using the
multiply_dictionary_values
function and prints the result.
Input/Output:
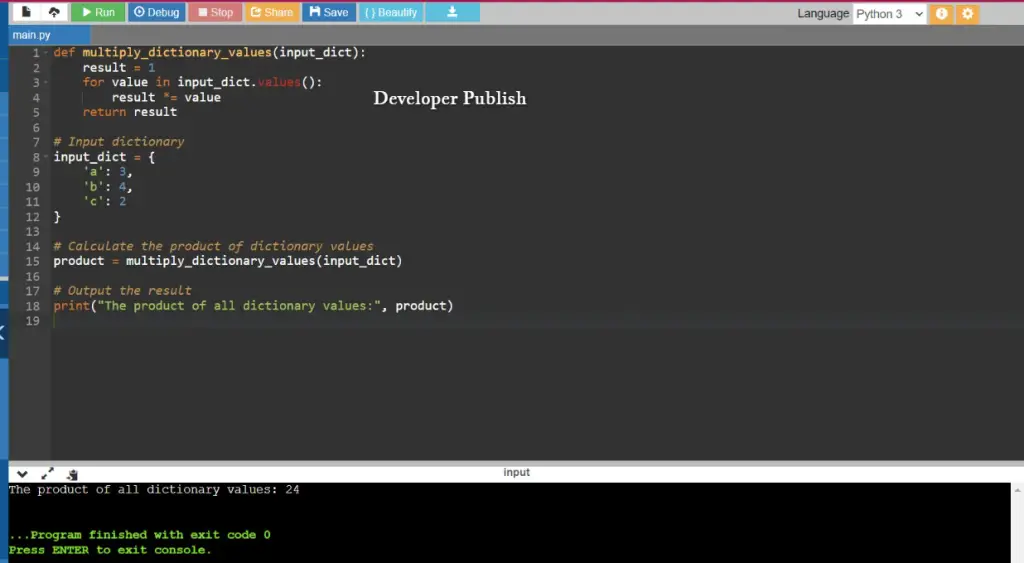