This python program calculates the sum of all nodes in a binary tree. Binary trees are hierarchical data structures commonly used in computer science to represent hierarchical relationships.
Problem statement
Given a binary tree, the task is to find the sum of all nodes present in the tree in python.
Python Program to Find the Sum of All Nodes in a Tree
class TreeNode: def __init__(self, value): self.value = value self.left = None self.right = None def sum_of_nodes(root): if root is None: return 0 return root.value + sum_of_nodes(root.left) + sum_of_nodes(root.right) root = TreeNode(5) root.left = TreeNode(3) root.right = TreeNode(8) root.left.left = TreeNode(1) root.left.right = TreeNode(4) root.right.left = TreeNode(6) root.right.right = TreeNode(9) total_sum = sum_of_nodes(root) print("Sum of all nodes in the tree:", total_sum)
How it works
- Node Definition: We define a class
TreeNode
representing a node in the binary tree. Each node contains a value and references to its left and right children. - Sum Calculation: We define a recursive function
sum_of_nodes(root)
that takes the root of the tree as its parameter and returns the sum of all node values in the tree. - Calculating the Sum: We call the
sum_of_nodes
function with the root of our example tree to calculate the sum of all node values: total_sum = sum_of_nodes(root) print(“Sum of all nodes in the tree:”, total_sum)
Input / Output
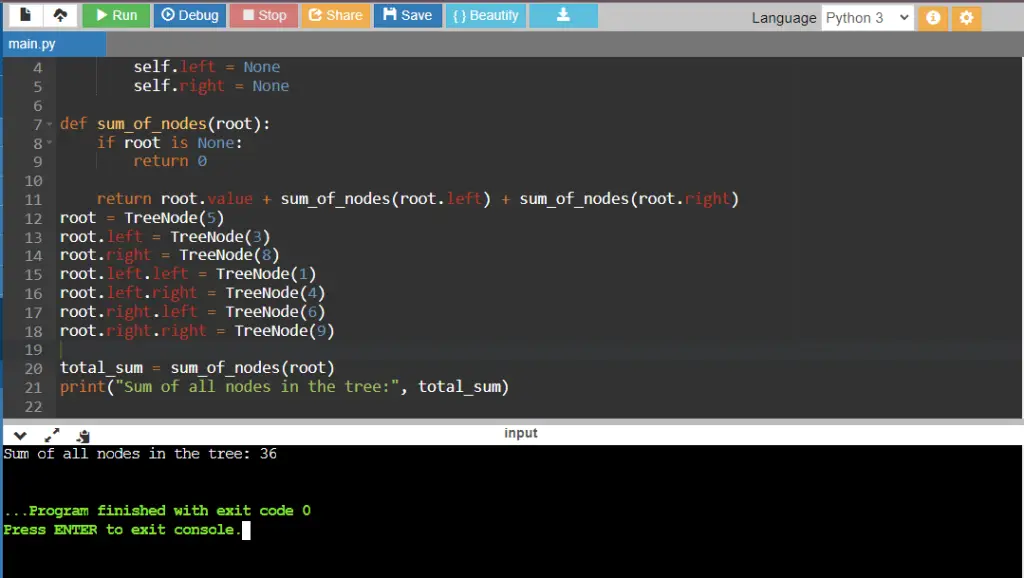