Bubble Sort is a simple sorting algorithm that repeatedly steps through the list, compares adjacent elements, and swaps them if they are in the wrong order. The pass through the list is repeated until the list is sorted. Although Bubble Sort is not the most efficient sorting algorithm, it’s easy to understand and implement.
Problem Statement
You are given a list of integers. Your task is to write a Python program to find the second largest number in the list using the Bubble Sort algorithm.
Python Program to Find the Second Largest Number in a List using Bubble Sort
def bubble_sort(arr): n = len(arr) for i in range(n): for j in range(0, n - i - 1): if arr[j] > arr[j + 1]: arr[j], arr[j + 1] = arr[j + 1], arr[j] def second_largest(arr): bubble_sort(arr) if len(arr) < 2: return None return arr[-2] # Example usage if __name__ == "__main__": try: input_list = [int(x) for x in input("Enter a list of numbers separated by spaces: ").split()] result = second_largest(input_list) if result is not None: print("The second largest number is:", result) else: print("List should have at least two numbers.") except ValueError: print("Invalid input. Please enter a valid list of numbers.")
How it Works
- Function Definitions:
bubble_sort(arr)
: This function implements the Bubble Sort algorithm. It iterates through the listarr
and compares adjacent elements. If an element is greater than its next element, they are swapped. This process is repeated for each element, causing the largest element to “bubble” to the end of the list.second_largest(arr)
: This function takes a listarr
as input, sorts it using thebubble_sort
function, and then returns the second-to-last element, which will be the second largest number in the list. If the list has fewer than two elements, the function returnsNone
.
- Example Usage Section:
- The program begins execution here when you run the script.
- It asks the user to input a list of numbers separated by spaces.
- It converts the input string into a list of integers using a list comprehension.
- It then calls the
second_largest
function with the input list. - If the result is not
None
, it prints the second largest number. If the list has fewer than two numbers, it prints an appropriate message.
- Bubble Sort Algorithm:
- The
bubble_sort
function sorts the input list using the Bubble Sort algorithm. - The outer loop runs
n
times, wheren
is the length of the list. - The inner loop compares adjacent elements and swaps them if they are in the wrong order. This loop runs
n - i - 1
times, wherei
is the current iteration of the outer loop. - After completing the Bubble Sort, the largest number will be at the last position in the list.
- The
- Finding the Second Largest:
- After sorting the list, the second largest number will be the element at the second-to-last position in the list.
- Output:
- The program outputs the second largest number in the list or appropriate messages if the list is empty or has fewer than two elements.
The program utilizes the Bubble Sort algorithm to sort the list and then extracts the second largest number from the sorted list.
Input/ Output
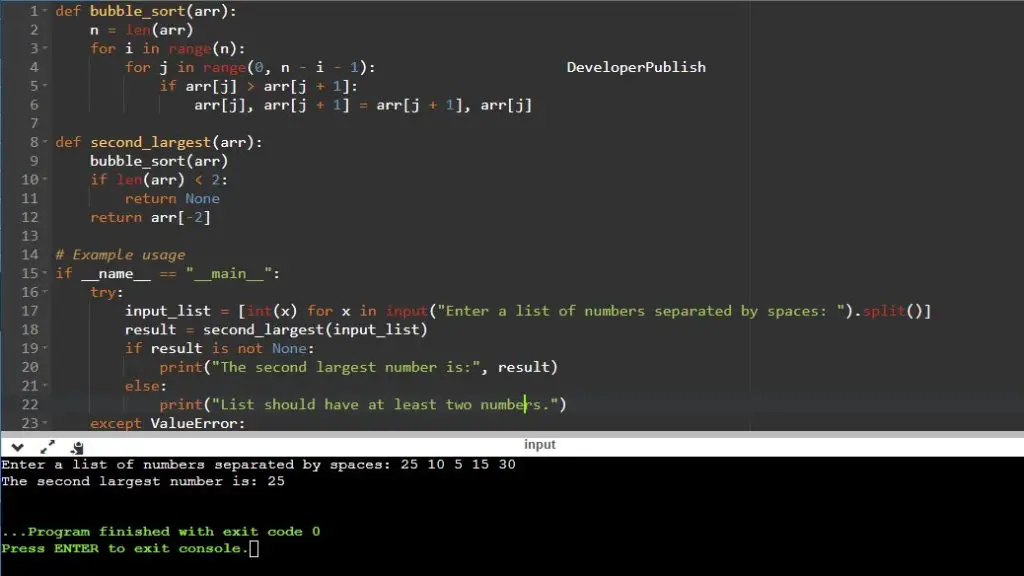