The provided Python program is designed to find the prime factors of a given number. It utilizes a function called prime_factors()
to accomplish this task. The program takes a number as input, either from the user or as a command-line argument, and proceeds to calculate the prime factors.
Problem Statement
Write a Python program that prompts the user to enter a positive integer and then finds and displays the prime factors of that number.
Requirements:
- The program should validate that the input is a positive integer. If the input is invalid, display an error message and prompt the user again until a valid input is provided.
- The program should calculate and display all the prime factors of the given number.
- The prime factors should be displayed in ascending order.
Python Program to Find the Prime Factors of a Number
def prime_factors(n): factors = [] i = 2 while i * i <= n: if n % i: i += 1 else: n //= i factors.append(i) if n > 1: factors.append(n) return factors # Example usage number = int(input("Enter a number: ")) factors = prime_factors(number) print("Prime factors of", number, "are:", factors)
How it Work
The Python program to find the prime factors of a given number works as follows:
- Prompt the user to enter a positive integer.
- Validate the input to ensure it is a positive integer. If the input is invalid (not a positive integer), display an error message and prompt the user again until a valid input is provided.
- Define a function called
prime_factors(n)
that takes the input numbern
as a parameter. - Initialize an empty list called
factors
to store the prime factors. - Initialize a variable
i
to 2. - Use a while loop that runs until
i * i
is greater thann
. This optimization ensures that we only check potential factors up to the square root ofn
. - Inside the while loop, check if
n
is divisible evenly byi
. If it is, dividen
byi
, appendi
to thefactors
list, and continue to the next iteration of the loop. - If
n
is not divisible byi
, incrementi
by 1 and continue to the next iteration of the loop. - After the while loop, check if
n
is greater than 1. If it is, it meansn
itself is a prime number, so append it to thefactors
list. - Return the
factors
list. - Call the
prime_factors()
function with the input number and store the result in a variable. - Print the prime factors of the given number.
The program follows this algorithm to find the prime factors of the input number. It utilizes a while loop, division, and iteration to determine the prime factors. The output is then displayed to the user.
Input/ Output
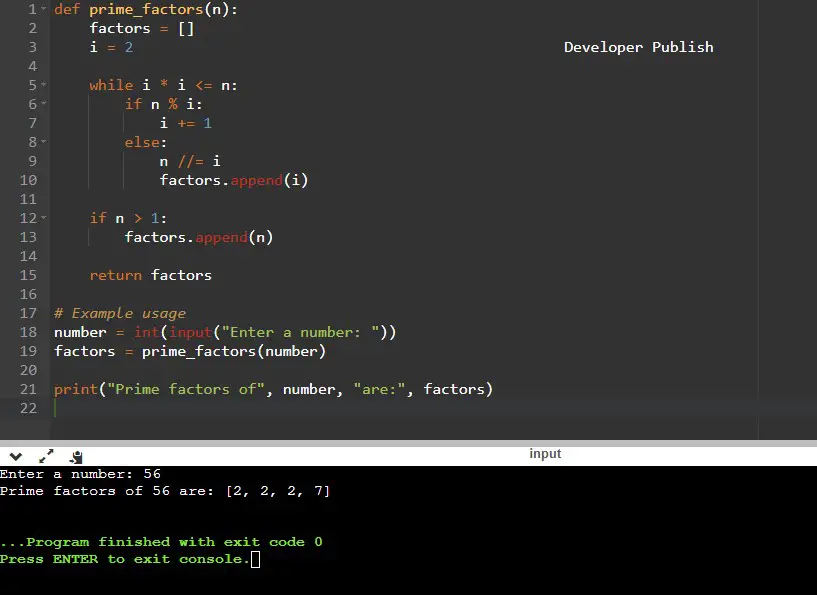
Explanation: The user enters the number 56. The prime factors of 56 are 2, 2, 2, and 7. The program calculates and displays the prime factors in ascending order.