In this tutorial, we will learn how to create a Python program that counts the occurrences of a given letter in a string using a recursive approach. Recursion is a technique where a function calls itself to solve a smaller instance of a problem.
Program Statement:
We want to write a Python program that takes a string and a letter as input and recursively determines how many times the given letter occurs in the string.
Python program to find the length of a given string without using any built in library functions
# Get the input string from the user input_string = input("Enter a string: ") # Initialize a variable to count the character length = 0 # Iterate through each character in the string for char in input_string: length += 1 # Print the length of the string print("Length of the string:", length)
How It Works:
The `count_letter_occurrences` function takes two parameters: `s` (the remaining string to process) and `letter` (the letter to count).
In the base case, if the string `s` is empty, the function returns 0, indicating no occurrences of the letter in the string.
In the recursive case, the function checks if the first character of the string `s` matches the given letter. If it does, it adds 1 to the result and calls itself recursively with the remaining string (`s[1:]`).-
If the first character doesn’t match the letter, the function simply calls itself with the remaining string (`s[1:]`).-
The recursion continues until the base case is reached, and all occurrences of the letter are counted.
Input/Output:
Enter a string: hello world
Length of the string : 11
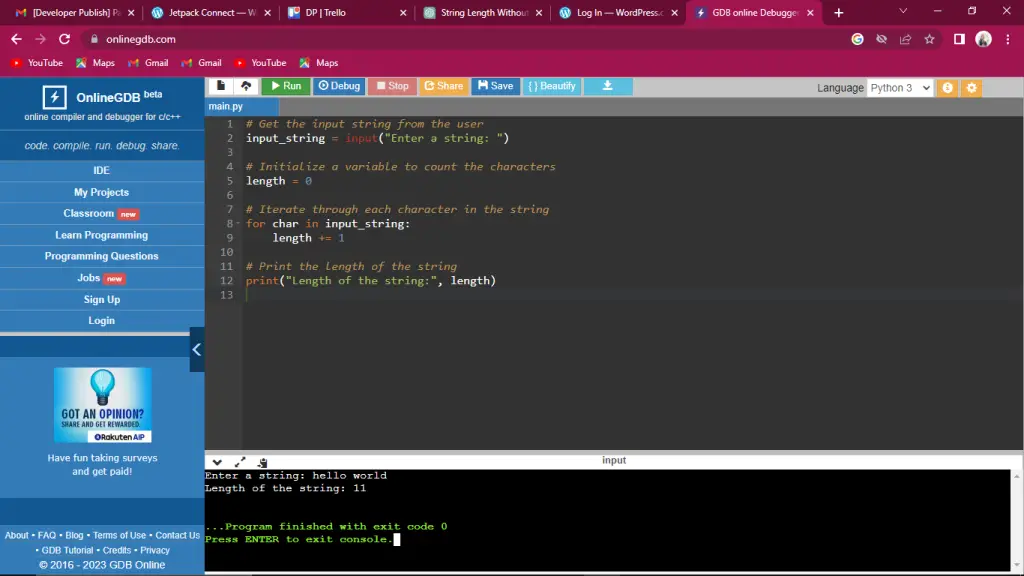