Python’s versatility shines when it comes to solving various programming challenges. In this example, we’ll create a Python program to find the cumulative sum of a given list of numbers.
Problem Statement
Write a Python program to calculate and display the cumulative sum of a given list. Given an input list, your program should generate an output list where each element represents the cumulative sum of all preceding elements, including itself.
Python Program to Find the Cumulative Sum of a List
def cumulative_sum(input_list): cumulative = [] current_sum = 0 for num in input_list: current_sum += num cumulative.append(current_sum) return cumulative # Input and Output input_list = [3, 5, 2, 8, 10] result = cumulative_sum(input_list) print("Input List:", input_list) print("Cumulative Sum List:", result)
Input / Output
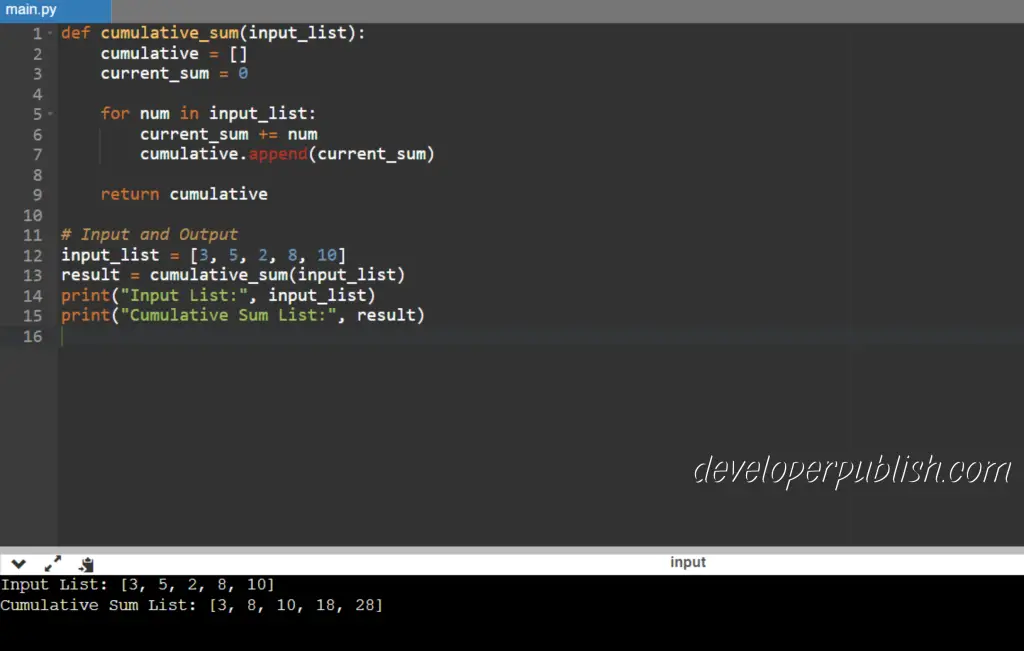