Palindrome detection is a common problem in computer science, where a palindrome is a string or sequence of elements that reads the same forwards as it does backwards. This python program addresses the task of checking whether a singly linked list is a palindrome or not.
Problem statement
Given a singly linked list, your goal is to determine whether the list is a palindrome. A singly linked list is considered a palindrome if the sequence of elements remains the same when read from the beginning to the end as well as from the end to the beginning.
Python Program to Check if Singly Linked List is Palindrome
class Node: def __init__(self, data): self.data = data self.next = None class LinkedList: def __init__(self): self.head = None def append(self, data): new_node = Node(data) if not self.head: self.head = new_node else: current = self.head while current.next: current = current.next current.next = new_node def is_palindrome(self): elements = [] current = self.head while current: elements.append(current.data) current = current.next return elements == elements[::-1] # Input: Creating a linked list linked_list = LinkedList() elements = [1, 2, 3, 2, 1] for element in elements: linked_list.append(element) print("Linked List:") current = linked_list.head while current: print(current.data, end=" -> ") current = current.next print("None") if linked_list.is_palindrome(): print("\nThe linked list is a palindrome.") else: print("\nThe linked list is not a palindrome.")
Input / Output
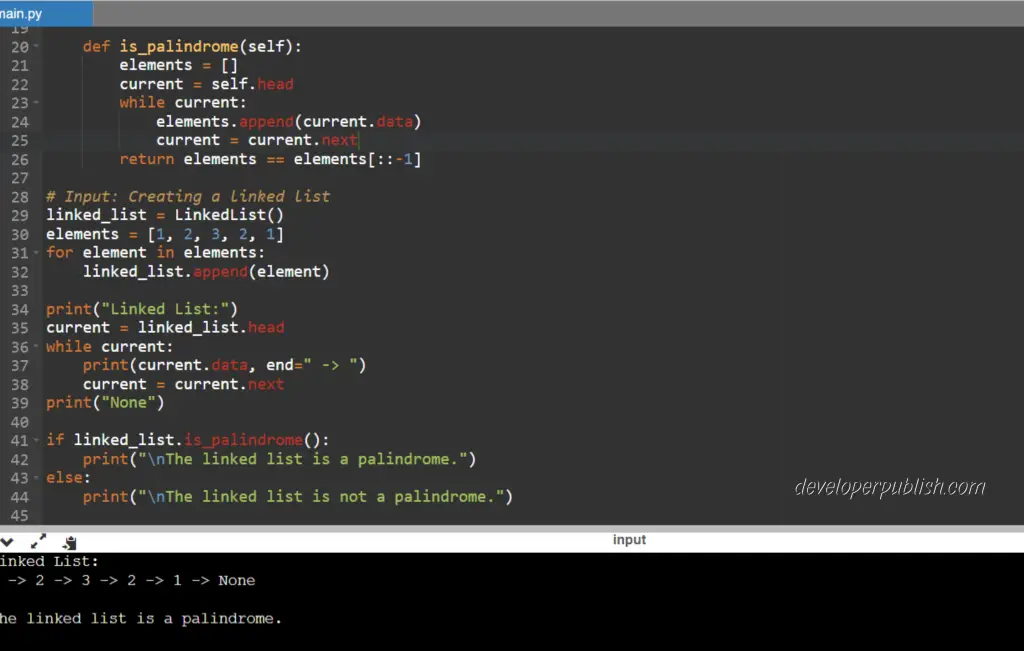