In this program, we will create a Python program to calculate the area of a triangle. The area of a triangle can be determined using its base and height. By taking user input for the base and height, we will calculate and display the area of the triangle using a simple formula.
Problem statement
Write a Python program to calculate the area of a triangle using the base and height provided by the user.
Python Program to Find the Area of a Triangle
# Python Program to Find the Area of a Triangle # Function to calculate the area of a triangle def calculate_area(base, height): area = (base * height) / 2 return area # Take user input for base and height base = float(input("Enter the base of the triangle: ")) height = float(input("Enter the height of the triangle: ")) # Calculate and display the area of the triangle area = calculate_area(base, height) print("The area of the triangle is:", area)
Input/Output
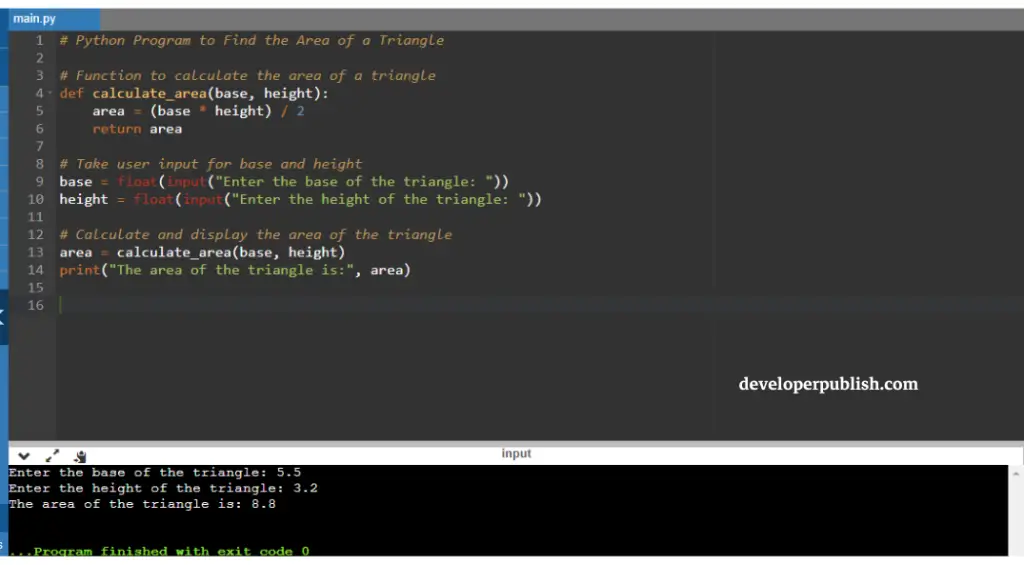