This Python program calculates the product of two numbers using recursion.
Recursion is a powerful programming technique where a function calls itself to solve a smaller instance of the same problem. It allows for elegant and concise solutions to certain types of problems. In Python, you can implement recursion by defining a function that calls itself within its body.
Program Statement
Write a Python program that calculates the product of two numbers using recursion. The program should prompt the user to enter two numbers and then use a recursive function to find their product. The program should display the result to the user.
The program should adhere to the following specifications:
- Define a function
multiply_recursive(a, b)
that takes two numbersa
andb
as parameters. - Implement the
multiply_recursive
function using recursion to find the product ofa
andb
. - Handle the base case: if either
a
orb
is 0, the product should be 0. - In the recursive case, multiply
a
by(b - 1)
and adda
to the result. This is equivalent to the formulaa * b = a + a * (b - 1)
. - Prompt the user to enter the first number (
num1
) and the second number (num2
). - Call the
multiply_recursive
function withnum1
andnum2
as arguments to calculate their product. - Display the product of the two numbers to the user.
Ensure that the program is able to handle positive integers, zero, and negative integers as input.
Python Program to Find Product of Two Numbers using Recursion
def multiply_recursive(a, b): # Base case: if either number is 0, the product is 0 if a == 0 or b == 0: return 0 # Recursive case: multiply a by b-1 and add a to the result # This is equivalent to a * b = a + a * (b - 1) return a + multiply_recursive(a, b - 1) # Test the function num1 = 4 num2 = 5 product = multiply_recursive(num1, num2) print(f"The product of {num1} and {num2} is: {product}")
How it works
- The program starts by defining a recursive function
multiply_recursive(a, b)
. This function takes two parametersa
andb
, representing the two numbers for which we want to calculate the product. - Inside the
multiply_recursive
function, we have a base case that checks if eithera
orb
is 0. If either number is 0, the product is also 0, so we return 0. - In the recursive case, we multiply
a
by(b - 1)
and adda
to the result. This step corresponds to the formulaa * b = a + a * (b - 1)
. By recursively callingmultiply_recursive(a, b - 1)
, we break down the problem into smaller subproblems until we reach the base case. - The program prompts the user to enter the first number (
num1
) and the second number (num2
) using theinput()
function. Theint()
function is used to convert the input strings to integers. - The
multiply_recursive
function is then called withnum1
andnum2
as arguments, and the result is assigned to the variableproduct
. - Finally, the program displays the product of the two numbers using
print()
, along with a formatted string that includes the input numbers and the calculated product.
When you run the program, it follows these steps to calculate the product of the two numbers using recursion. The recursion occurs as the function repeatedly calls itself with smaller values of b
until it reaches the base case. Once the base case is reached, the recursive calls start returning their values, and the product is calculated.
Input/Output
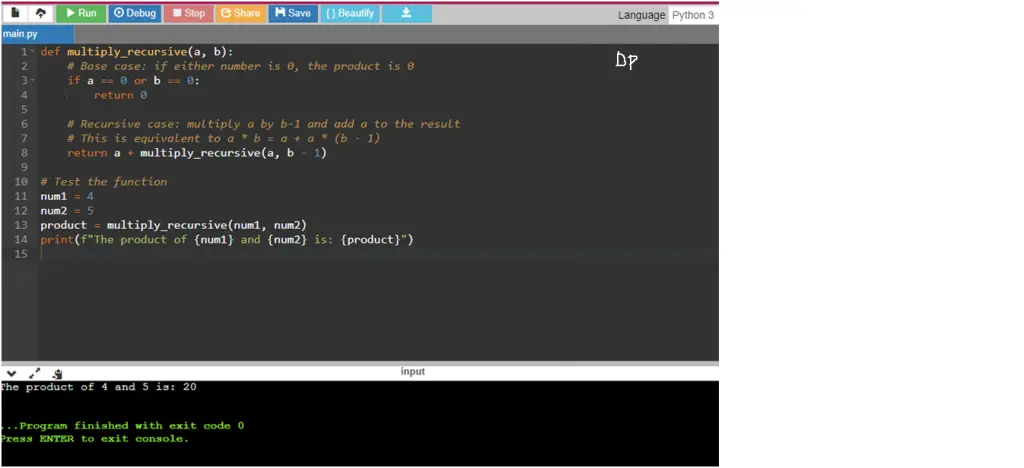