In this Python program, we will leverage recursion to count the occurrences of elements within a linked list. This program will provide you with a deeper understanding of recursion and how it can be applied to solve problems involving linked lists.
Problem statement
The challenge is to write a Python program that counts the occurrences of elements in a given linked list using recursion. By employing recursion, you can efficiently traverse the linked list and tally the number of occurrences of each element.
Python Program to Count the Occurrences of Elements in a Linked List using Recursion
class Node: def __init__(self, data): self.data = data self.next = None class LinkedList: def __init__(self): self.head = None def insert(self, data): new_node = Node(data) if not self.head: self.head = new_node else: current = self.head while current.next: current = current.next current.next = new_node def count_occurrences(self, head, target): if not head: return 0 if head.data == target: return 1 + self.count_occurrences(head.next, target) else: return self.count_occurrences(head.next, target) # Example usage if __name__ == "__main__": linked_list = LinkedList() elements = [2, 4, 3, 4, 2, 5, 4] for element in elements: linked_list.insert(element) target_element = 4 occurrences = linked_list.count_occurrences(linked_list.head, target_element) print(f"Occurrences of {target_element}: {occurrences}")
Input / Output
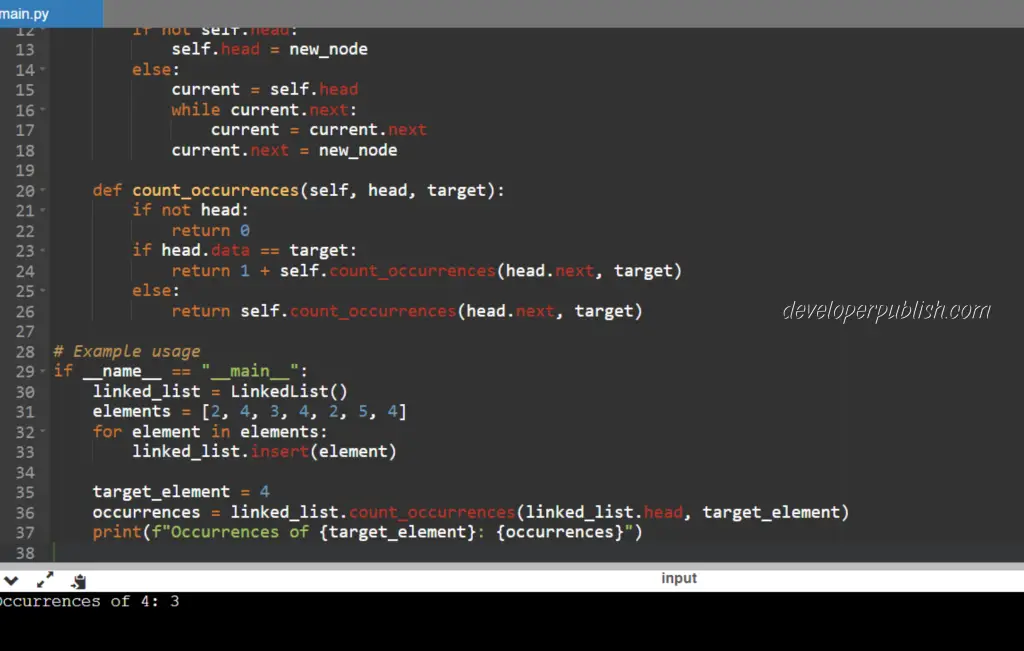