In this Python program, we’ll walk you through a straightforward method to count how many times a particular element appears in a given list. We’ll use a simple function to accomplish this, and by the end, you’ll have a clear understanding of how to perform this task on your own lists.
Problem statement
You are tasked with developing a Python program that can count the number of times a specific element appears in a given list. This program should be versatile, allowing users to input their own lists and specify which element they want to count.
Python Program to Count Occurrences of Element in List
def count_occurrences(lst, element_to_count): count = 0 for item in lst: if item == element_to_count: count += 1 return count # Example usage: my_list = [1, 2, 3, 4, 2, 2, 3, 1, 1] element = 2 occurrences = count_occurrences(my_list, element) print(f"The element {element} occurs {occurrences} times in the list.")
How it works
Sure, let’s break down how the Python program works to count the occurrences of an element in a list based on the provided problem statement:
- Defining the Function
count_occurrences
:- The program defines a function called
count_occurrences
that takes two arguments:lst
(the list in which we want to count occurrences) andelement_to_count
(the element we want to count).
- The program defines a function called
- Initializing a Count Variable:
- Inside the
count_occurrences
function, a variable calledcount
is initialized to zero. This variable will be used to keep track of the number of occurrences ofelement_to_count
in the list.
- Inside the
- Iterating Through the List:
- The program uses a loop (typically a
for
loop) to iterate through each item in the input listlst
. It examines each element one by one.
- The program uses a loop (typically a
- Comparing Elements:
- Within the loop, the program compares the current element being examined with the
element_to_count
. If they match (i.e., if the current element is equal toelement_to_count
), it increments thecount
variable by 1.
- Within the loop, the program compares the current element being examined with the
- Returning the Count:
- After the loop has iterated through the entire list, the
count
variable contains the total count of occurrences ofelement_to_count
in the list. The function returns this count as the result.
- After the loop has iterated through the entire list, the
- User Input and Output:
- Outside the
count_occurrences
function, the program provides a user-friendly interface where the user can input their own list and the element they want to count. - It calls the
count_occurrences
function with the user’s inputs and displays the count of occurrences to the user. - The program should handle situations where the specified element may not be present in the list and inform the user accordingly.
- Outside the
- Documentation and Comments:
- The program is well-documented, with clear comments explaining each part of the code. This makes it easier for others (and the programmer) to understand how the program works.
- Example Execution:
- An example execution of the program is provided in the problem statement, demonstrating how the user interacts with the program and how it counts the occurrences of the specified element in the given list.
Overall, the program combines user input, looping, conditional checks, and function definitions to efficiently count the occurrences of an element in a list and provide the result to the user.
Input/Output
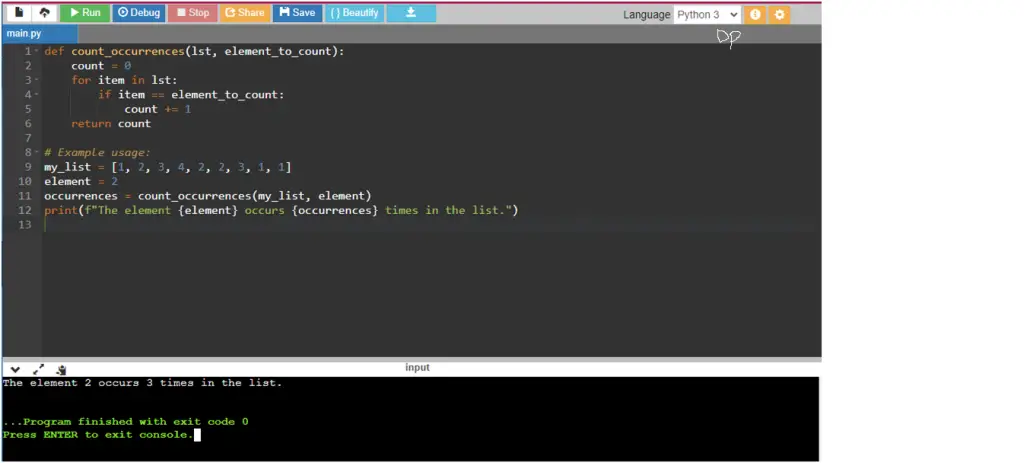