This Python program counts the number of lowercase characters in a string.
Problem Statement
You are tasked with writing a Python program that counts the number of lowercase characters in a given string.
Python Program to Count Number of Lowercase Characters in a String
# Function to count lowercase characters in a string def count_lowercase_characters(input_string): count = 0 for char in input_string: if char.islower(): count += 1 return count # Input string input_string = input("Enter a string: ") # Call the function to count lowercase characters lowercase_count = count_lowercase_characters(input_string) # Display the result print("Number of lowercase characters:", lowercase_count)
How it works
Certainly! Here’s how the Python program to count the number of lowercase characters in a string works:
- User Input:
- The program starts by prompting the user to enter a string. This input is obtained using the
input()
function, which waits for the user to type in a string and press Enter.
- The program starts by prompting the user to enter a string. This input is obtained using the
- Function Definition:
- The program defines a function called
count_lowercase_characters(input_string)
. This function takes the user’s input string as its argument.
- The program defines a function called
- Counting Lowercase Characters:
- Inside the function, there is a variable called
count
initialized to 0. This variable will be used to keep track of the count of lowercase characters. - The program then enters a loop that iterates through each character in the input string one by one. This is done using a
for
loop. - For each character, it checks whether it is a lowercase character using the
islower()
method. If the character is lowercase, it increments thecount
variable by 1.
- Inside the function, there is a variable called
- Return Count:
- After looping through all the characters in the input string, the function returns the value of
count
, which represents the count of lowercase characters in the input string.
- After looping through all the characters in the input string, the function returns the value of
- Main Execution:
- Back in the main part of the program, the user’s input string is obtained.
- The
count_lowercase_characters()
function is called with the input string as its argument, and the result is stored in a variable calledlowercase_count
.
- Display Result:
- Finally, the program displays the result by printing the count of lowercase characters along with an informative message.
Here’s a step-by-step breakdown of the program’s execution:
- User enters a string, e.g., “Hello World.”
- The program calls
count_lowercase_characters("Hello World")
. - Inside the function, it counts the lowercase characters (‘e’, ‘l’, ‘l’, ‘o’, ‘o’, ‘r’, ‘l’, ‘d’) and returns 6.
- The program prints “Number of lowercase characters: 6” as the output.
This program effectively counts the lowercase characters in a given string and provides the count as the output.
Input/Output
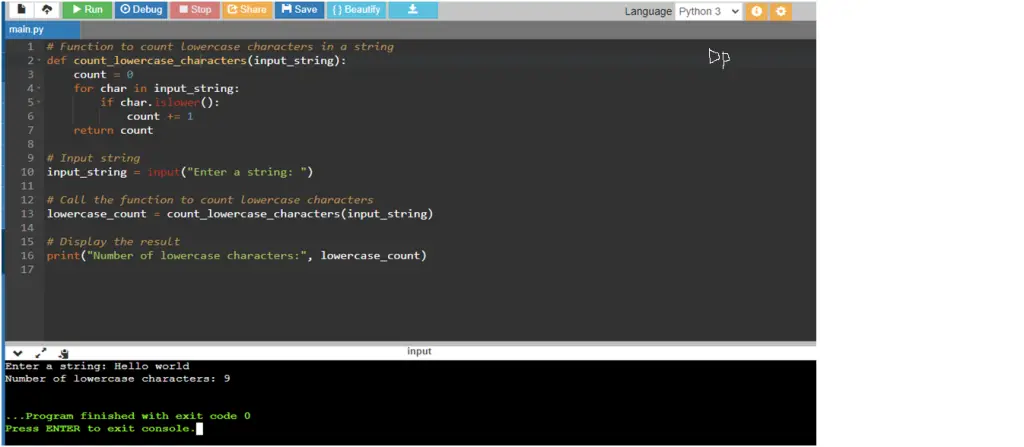