In this Python program, we will convert a binary number to its corresponding Gray code. The Gray code is a binary numeral system where two consecutive values differ by only one bit position. It finds applications in various fields, such as error detection and digital communications.
Problem Statement
Given a binary number as input, we need to convert it into its equivalent Gray code representation.
Python Program to Convert Binary to Gray Code
def binary_to_gray(binary): gray = binary[0] # The first bit of Gray code remains the same as the binary number for i in range(1, len(binary)): # XOR operation between consecutive bits of binary number gray += str(int(binary[i]) ^ int(binary[i - 1])) return gray # Main program binary_number = input("Enter a binary number: ") gray_code = binary_to_gray(binary_number) print("Gray code:", gray_code)
How It Works
- The
binary_to_gray()
function takes a binary number as input and returns its corresponding Gray code representation. - The first bit of the Gray code remains the same as the first bit of the binary number.
- For each subsequent bit in the binary number, we perform an XOR operation between the current bit and the previous bit in the binary number. The result of the XOR operation gives us the corresponding bit in the Gray code.
- We iterate through the binary number starting from the second bit (
i = 1
) and append the XOR result to the Gray code string. - Finally, we return the Gray code representation of the binary number.
- In the main program, we prompt the user to enter a binary number.
- We pass the binary number to the
binary_to_gray()
function to convert it to Gray code. - The resulting Gray code is then printed as output.
Input/Output
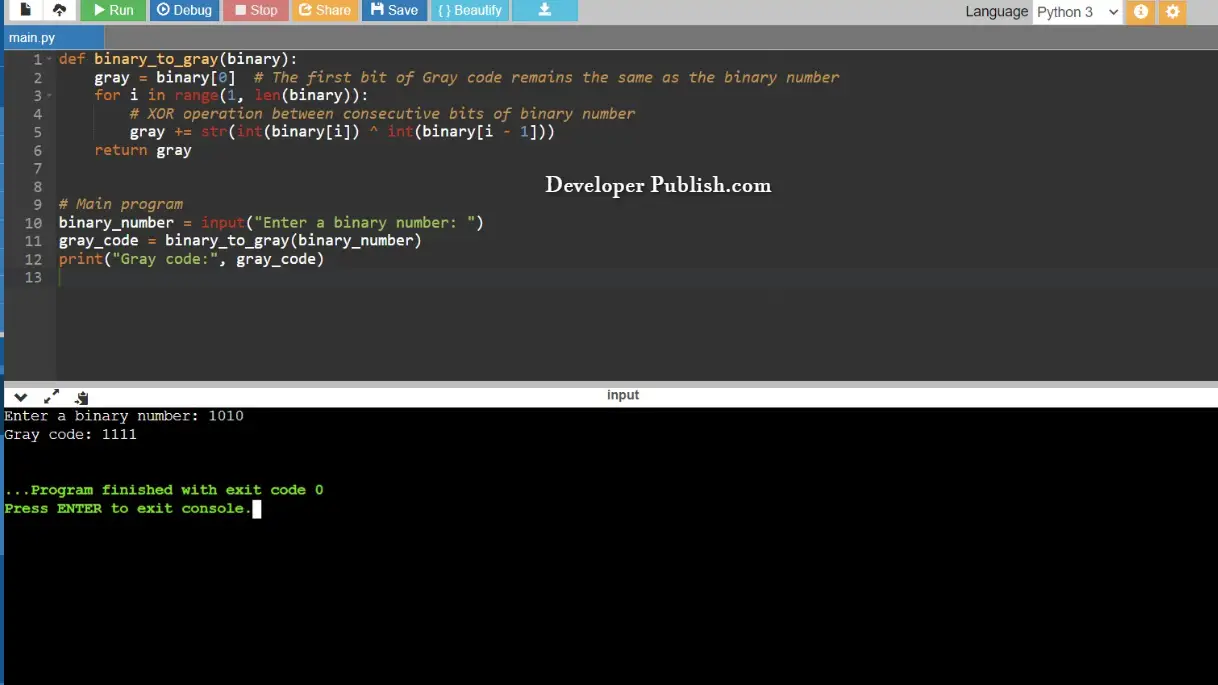