If you are using Dictionary and wondering how to sort it based on the value , you can pretty much do it easily using the lambda expression of linq in .NET.
How to Sort Dictionary by its Value in C# ?
Assume that you have the dictionary with the following items in it.
Dictionary<int, string> keyPairs = new Dictionary<int, string>(); keyPairs.Add(1, "one"); keyPairs.Add(2, "two"); keyPairs.Add(3, "three"); keyPairs.Add(4, "four");
If you want to sort it based on the values (string) , you can first convert this to a List and sort it using the lamba expression as shown belo.
var lst = keyPairs.ToList(); lst.Sort((pair1, pair2) => pair1.Value.CompareTo(pair2.Value));
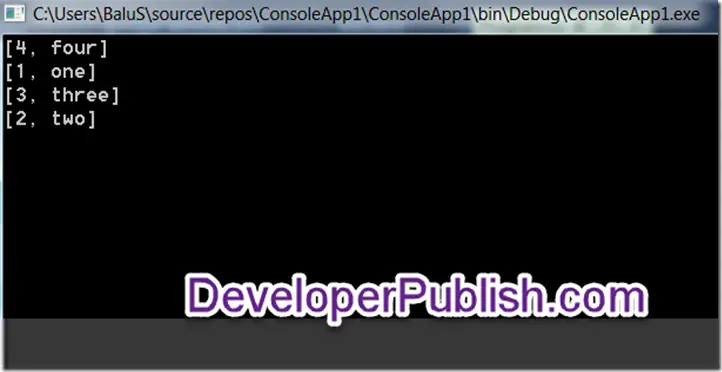
Here’s the full code snippet that is used for the demo in this blog post.
using System; using System.Linq; using System.Collections.Generic; namespace ConsoleApp1 { class Program { static void Main(string[] args) { Dictionary<int, string> keyPairs = new Dictionary<int, string>(); keyPairs.Add(1, "one"); keyPairs.Add(2, "two"); keyPairs.Add(3, "three"); keyPairs.Add(4, "four"); var lst = keyPairs.ToList(); lst.Sort((pair1, pair2) => pair1.Value.CompareTo(pair2.Value)); foreach(var item in lst) Console.WriteLine(item); Console.ReadLine(); } } }