This C# program prints the famous “Hello, World!” message to the console without using Console.WriteLine
.
Problem Statement
Write a Program in C# to Print Hello World Without using WriteLine
C# Program to Print Hello World Without using WriteLine
using System; class Program { static void Main() { Console.Write("Hello, World!"); } }
How it Works
Using the Console
Class:
- In C#, you can interact with the console (the command-line interface where you can input and output text) using the
Console
class, which is part of theSystem
namespace.
Console.Write Method:
- The
Console.Write
method is a part of theConsole
class, and it allows you to display text on the console without automatically adding a newline character.
Code Explanation:
- Here’s a breakdown of the C#
using System;
: This line is an import statement that brings in theSystem
namespace, which includes theConsole
class.class Program
: Defines a class named “Program,” which contains the program’s code.static void Main()
: This is the entry point of the program, where execution begins. It’s a special method in C# that serves as the program’s starting point.Console.Write("Hello, World!");
: Within theMain
method,Console.Write
is used to display the text “Hello, World!” on the console without adding a newline character. As a result, the text is printed on the same line.
Running the Program:
- When you compile and run this C# program, it will execute the
Main
method. - The
Console.Write
statement will display “Hello, World!” on the console without moving to the next line. - After displaying the text, the program will exit, and you’ll see the output on your console.
Input/ Output
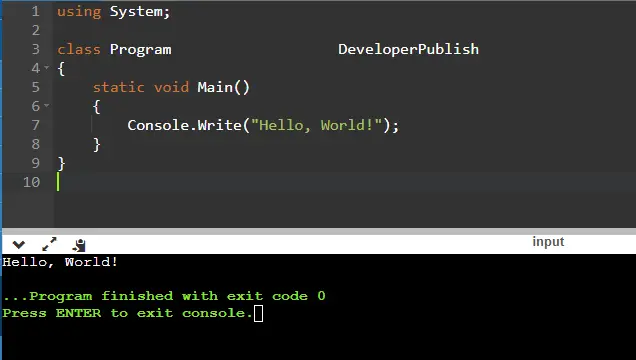