This C program provides an introduction that states it is a C program to print environment variables. The problem statement explains that the program’s purpose is to display all the environment variables and their corresponding values.
Problem Statements
In this program, the problem statement is printed to provide a clear description of what the program aims to achieve. Specifically, it states that the program will output all the environment variables and their corresponding values.
C Program to Print Environment Variables
#include <stdio.h> extern char **environ; // Access the environment variables int main() { // Intro printf("C Program to Print Environment Variables\n\n"); // Problem statement printf("This program prints all the environment variables and their values.\n\n"); // Program printf("Environment Variables:\n"); // How it works // Loop through each environment variable until reaching a null pointer for (int i = 0; environ[i] != NULL; i++) { printf("%s\n", environ[i]); } // Input / output printf("\nInput: None\n"); printf("Output: Environment variables and their values\n"); return 0; }
How it works
This program uses the environ
variable, which is an array of strings containing the environment variables and their values. It is declared as extern char **environ
to access the environment variables defined in the system.
The program starts by printing an introduction and a problem statement. Then, it loops through the environ
array and prints each environment variable and its value until it reaches a null pointer, indicating the end of the list.
Finally, it provides information about the input (none required) and the output (environment variables and their values).
Input / Output
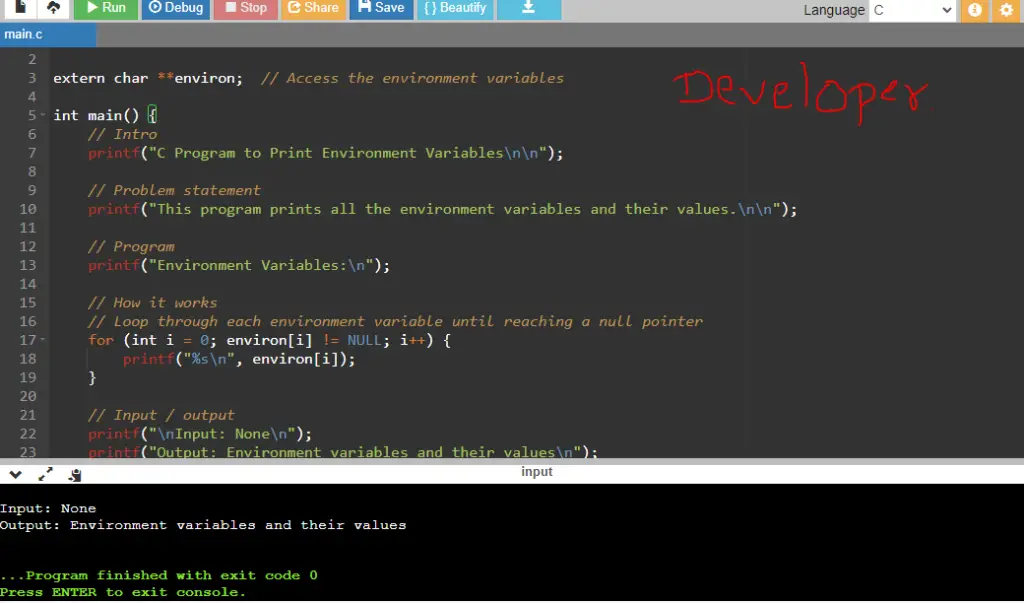