Introduction
This C program prints a diamond pattern based on the number of rows provided by the user. It uses nested loops to iterate through each row and column, printing spaces and asterisks accordingly.
Problem statement
Write a C program to print a diamond pattern based on the number of rows provided by the user.
C Program to Print Diamond Pattern
#include <stdio.h> void printDiamondPattern(int n) { int i, j, space; // Print upper half of the diamond for (i = 1; i <= n; i++) { // Print spaces for (space = 1; space <= n - i; space++) { printf(" "); } // Print asterisks for (j = 1; j <= 2 * i - 1; j++) { printf("*"); } printf("\n"); } // Print lower half of the diamond for (i = n - 1; i >= 1; i--) { // Print spaces for (space = 1; space <= n - i; space++) { printf(" "); } // Print asterisks for (j = 1; j <= 2 * i - 1; j++) { printf("*"); } printf("\n"); } } int main() { int n; printf("Enter the number of rows (odd number): "); scanf("%d", &n); // Check if the number of rows is odd if (n % 2 == 0) { printf("Invalid input! Number of rows should be odd.\n"); return 0; } printDiamondPattern(n); return 0; }
How it works?
- The program prompts the user to enter the number of rows for the diamond pattern (should be an odd number).
- The input is stored in the variable
n
. - The program checks if the number of rows is odd. If it is even, an error message is displayed and the program terminates.
- The function
printDiamondPattern()
is called with the value ofn
. - Inside the
printDiamondPattern()
function, two nested loops are used to print the upper half and lower half of the diamond pattern. - In each loop iteration, the appropriate number of spaces and asterisks is printed based on the current row and column.
- After printing all the rows, the program terminates.
Input/Output
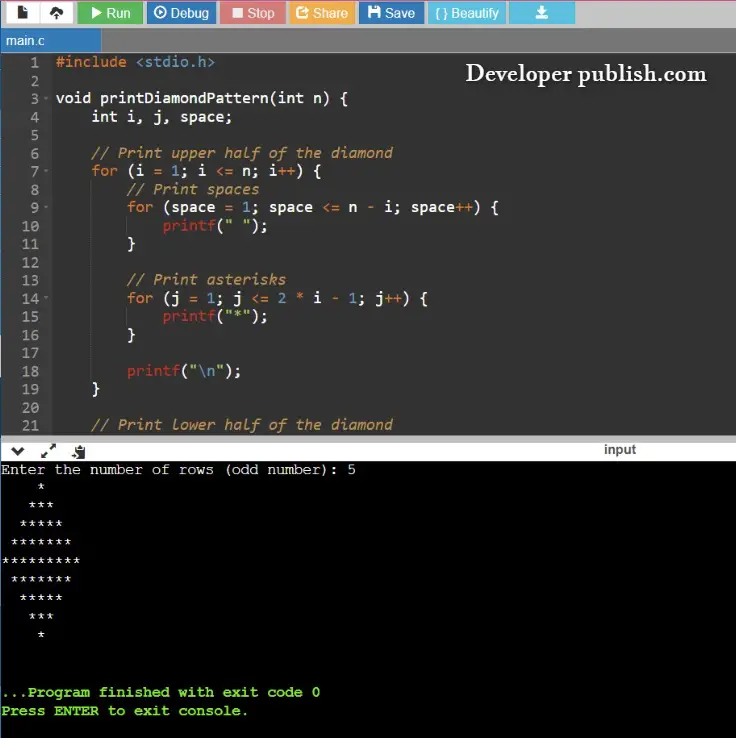