This C program finds and prints all non-repeated elements in an array. It provides a solution to identify unique elements without repetition.
Program Statement
Program Statement: Print all Non-Repeated Elements in an Array
Write a C program that reads an array of integers and prints all the non-repeated elements present in the array. The program should implement the following:
- Prompt the user to enter the size of the array.
- Read the array elements from the user.
- Define a function
printNonRepeatedElements
that takes the array and its size as parameters. - Inside the
printNonRepeatedElements
function, iterate through each element of the array. - For each element, count the number of occurrences by comparing it with all other elements in the array.
- If the count is equal to 1, print the element as a non-repeated element.
- In the
main
function, call theprintNonRepeatedElements
function with the array and its size. - Print the non-repeated elements obtained from the
printNonRepeatedElements
function.
Ensure that your program handles different test cases and works correctly for arrays of varying sizes.
C Program to Print all Non Repeated Elements in an Array
#include <stdio.h> void printNonRepeatedElements(int arr[], int size) { // Iterate through each element in the array for (int i = 0; i < size; i++) { int count = 0; // Check if the element is repeated for (int j = 0; j < size; j++) { if (arr[i] == arr[j]) count++; } // If the count is 1, the element is non-repeated if (count == 1) printf("%d ", arr[i]); } } int main() { int arr[] = {2, 4, 5, 2, 6, 7, 8, 6, 4}; int size = sizeof(arr) / sizeof(arr[0]); printf("Non-repeated elements in the array: "); printNonRepeatedElements(arr, size); return 0; }
How it Works?
- The program starts by prompting the user to enter the size of the array.
- The user enters the size of the array, and the program reads it.
- The program then prompts the user to enter the elements of the array.
- The user enters the elements of the array, and the program reads them.
- The program defines a function called
printNonRepeatedElements
that takes the array and its size as parameters. - Inside the
printNonRepeatedElements
function, the program iterates through each element of the array using a loop. - For each element, the program initializes a variable called
count
to 0. This variable will be used to count the number of occurrences of the current element. - The program then uses another loop to compare the current element with all other elements in the array.
- If the current element matches with another element in the array, the
count
variable is incremented. - After counting the occurrences of the current element, the program checks if the
count
is equal to 1. - If the
count
is 1, it means that the current element is non-repeated because it only occurred once in the array. - In such a case, the program prints the current element as a non-repeated element.
- Once the
printNonRepeatedElements
function has iterated through all the elements in the array, it returns to themain
function. - In the
main
function, the program calls theprintNonRepeatedElements
function with the array and its size as arguments. - The
printNonRepeatedElements
function processes the array and finds the non-repeated elements. - The non-repeated elements obtained from the
printNonRepeatedElements
function are printed in themain
function. - The program ends.
By following these steps, the program identifies and prints all the non-repeated elements present in the given array.
Input/Output
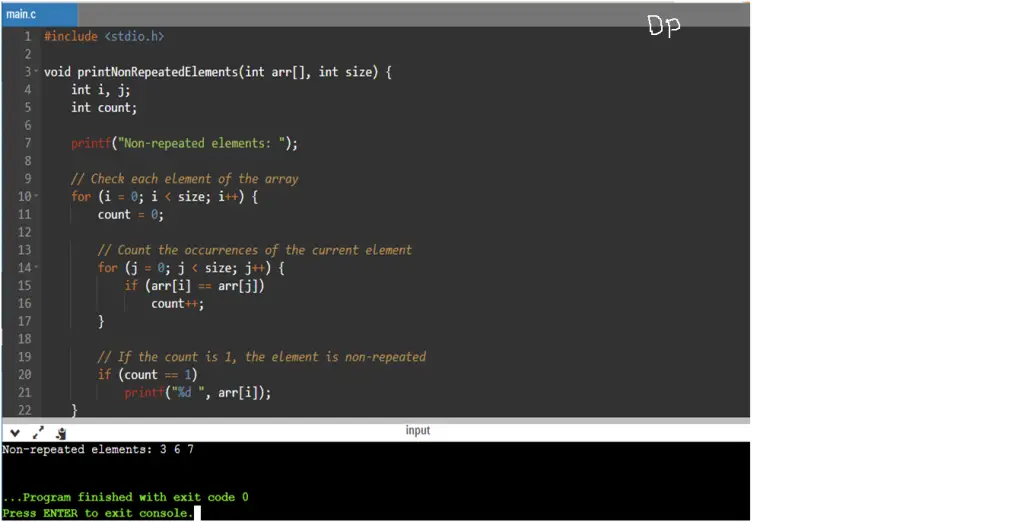