This C program calculates the volume and surface area of a cone based on the provided radius and height.
Problem statement
Given the radius and height of a cone, the program calculates its volume and surface area.
The program follows the steps below:
- It prompts the user to enter the radius and height of the cone.
- The program calculates the slant height of the cone using the Pythagorean theorem.
- Using the provided inputs and calculated slant height, the program computes the volume and surface area of the cone.
- Finally, it displays the calculated volume and surface area on the screen.
C Program to Find Volume and Surface Area of Cone
#include<stdio.h> #include<math.h> int main() { float radius, height; float volume, surface_area; float slant_height; printf("***** Cone Volume and Surface Area Calculator *****\n\n"); // Input radius and height of the cone printf("Enter the radius of the cone: "); scanf("%f", &radius); printf("Enter the height of the cone: "); scanf("%f", &height); // Calculate the slant height slant_height = sqrt(pow(radius, 2) + pow(height, 2)); // Calculate the volume of the cone volume = (1.0 / 3.0) * M_PI * pow(radius, 2) * height; // Calculate the surface area of the cone surface_area = M_PI * radius * (radius + slant_height); printf("\nVolume of the cone: %.2f cubic units\n", volume); printf("Surface area of the cone: %.2f square units\n", surface_area); return 0; }
How it works
- The program prompts the user to enter the radius and height of the cone.
- The program calculates the slant height of the cone using the formula
sqrt(radius^2 + height^2)
. - Using the provided inputs and calculated slant height, the program calculates the volume using the formula
(1/3) * π * radius^2 * height
and the surface area using the formulaπ * radius * (radius + slant_height)
. - The calculated volume and surface area are displayed on the screen
Input / output
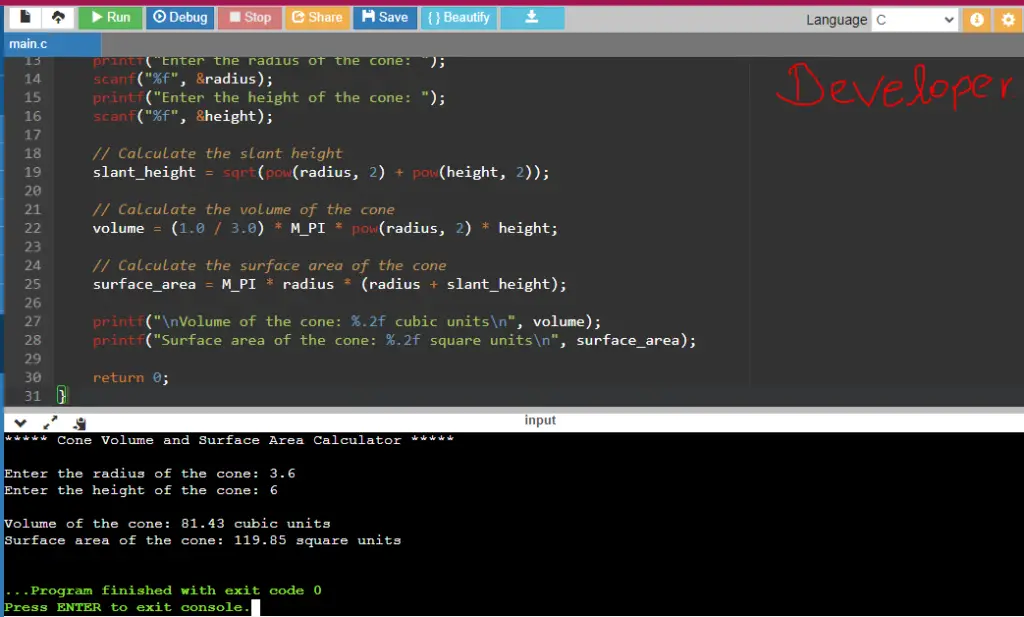