In statistics, the standard deviation is a measure of the amount of variation or dispersion of a set of values. In this task, we will write a C# program to find the standard deviation of a set of numbers.
Problem Statement
Write a C# program to find the standard deviation of a set of numbers. The program should take input from the user in the form of a set of numbers. The program should display the standard deviation of the numbers as output.
C# Program to Find the Standard Deviation
using System; using System.Collections.Generic; using System.Linq; class Program { static void Main(string[] args) { Console.WriteLine("Enter the numbers separated by spaces: "); string input = Console.ReadLine(); string[] numbers = input.Split(' '); List<double> values = new List<double>(); foreach (string number in numbers) { values.Add(double.Parse(number)); } double mean = values.Average(); double sumOfSquares = 0; foreach (double value in values) { sumOfSquares += Math.Pow(value - mean, 2); } double standardDeviation = Math.Sqrt(sumOfSquares / values.Count); Console.WriteLine("Standard Deviation: {0}", standardDeviation); } }
Input / Output
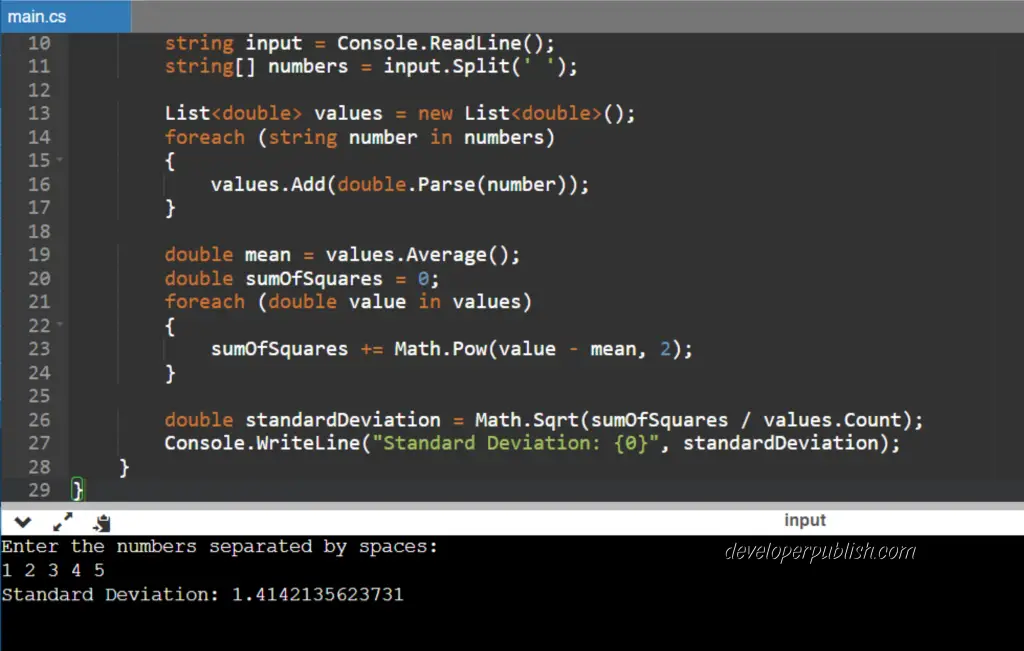