This C program calculates and compares the perimeters of three different geometric shapes: a circle, a rectangle, and a triangle. Perimeter is the total length of the boundary of a shape, and it varies depending on the specific attributes of each shape.
Problem Statement
Write a C program that calculates the perimeter of different shapes, including a circle, rectangle, and triangle. The program should provide a menu for the user to select the shape and then prompt for the required dimensions to calculate the perimeter.
The program should perform the following tasks:
- Display an introduction to the program.
- Present a menu with the following options:
- Circle
- Prompt the user to enter their choice (1-4) from the menu.
- Based on the user’s choice, perform the following tasks:
- If the user selects 1 (Circle):
- Prompt the user to enter the radius of the circle.
- Calculate the perimeter of the circle using the formula: perimeter = 2 * PI * radius, where PI is a constant value (3.14159265).
- Display the calculated perimeter of the circle.
- If the user selects 2 (Rectangle):
- Prompt the user to enter the length and width of the rectangle.
- Calculate the perimeter of the rectangle using the formula: perimeter = 2 * (length + width).
- Display the calculated perimeter of the rectangle.
- If the user selects 3 (Triangle):
- Prompt the user to enter the lengths of the three sides of the triangle.
- Calculate the perimeter of the triangle by adding the lengths of the three sides.
- Display the calculated perimeter of the triangle.
- If the user selects 4 (Exit):
- End the program.
- If the user selects an invalid choice (other than 1-4):
- Display an error message indicating an invalid choice.
- If the user selects 1 (Circle):
- After displaying the perimeter, return to the menu to allow the user to perform additional calculations or exit the program.
C Program to Find the Perimeter of a Circle, Rectangle and Triangle
#include <stdio.h> #define PI 3.14159265 float calculateCirclePerimeter(float radius) { return 2 * PI * radius; } float calculateRectanglePerimeter(float length, float width) { return 2 * (length + width); } float calculateTrianglePerimeter(float side1, float side2, float side3) { return side1 + side2 + side3; } int main() { printf("Perimeter Calculation Program\n"); printf("=============================\n\n"); int choice; printf("Select the shape for which you want to calculate the perimeter:\n"); printf("1. Circle\n"); printf("2. Rectangle\n"); printf("3. Triangle\n"); printf("Enter your choice (1-3): "); scanf("%d", &choice); float radius, length, width, side1, side2, side3; switch (choice) { case 1: printf("Enter the radius of the circle: "); scanf("%f", &radius); float circlePerimeter = calculateCirclePerimeter(radius); printf("Perimeter of the circle: %.2f\n", circlePerimeter); break; case 2: printf("Enter the length and width of the rectangle: "); scanf("%f %f", &length, &width); float rectanglePerimeter = calculateRectanglePerimeter(length, width); printf("Perimeter of the rectangle: %.2f\n", rectanglePerimeter); break; case 3: printf("Enter the lengths of the three sides of the triangle: "); scanf("%f %f %f", &side1, &side2, &side3); float trianglePerimeter = calculateTrianglePerimeter(side1, side2, side3); printf("Perimeter of the triangle: %.2f\n", trianglePerimeter); break; default: printf("Invalid choice!\n"); break; } return 0; }
How it Works
The C program to find the perimeter of a circle, rectangle, and triangle works as follows:
- The program begins by displaying an introduction to the program and providing a menu of shape options (circle, rectangle, triangle, and exit).
- The user is prompted to enter their choice by inputting a number corresponding to the desired shape.
- Based on the user’s choice, the program executes the corresponding case in a switch statement.
- If the user selects 1 (Circle), the program prompts the user to enter the radius of the circle.
- The program then calls the
calculateCirclePerimeter
function, passing the radius as an argument.- The
calculateCirclePerimeter
function multiplies the radius by 2 and PI (a constant value) to calculate the perimeter of the circle. - The calculated perimeter is returned to the main function.
- The
- The program displays the calculated perimeter of the circle.
- If the user selects 2 (Rectangle), the program prompts the user to enter the length and width of the rectangle.
- The program then calls the
calculateRectanglePerimeter
function, passing the length and width as arguments.- The
calculateRectanglePerimeter
function adds the length and width, multiplies the sum by 2, and calculates the perimeter of the rectangle. - The calculated perimeter is returned to the main function.
- The
- The program displays the calculated perimeter of the rectangle.
- If the user selects 3 (Triangle), the program prompts the user to enter the lengths of the three sides of the triangle.
- The program then calls the
calculateTrianglePerimeter
function, passing the side lengths as arguments.- The
calculateTrianglePerimeter
function adds the three side lengths to calculate the perimeter of the triangle. - The calculated perimeter is returned to the main function.
- The
- The program displays the calculated perimeter of the triangle.
- If the user selects 4 (Exit), the program terminates.
- If the user selects an invalid choice (other than 1-4), the program displays an error message indicating an invalid choice.
- After displaying the perimeter, the program returns to the menu to allow the user to perform additional calculations or exit the program.
The program uses functions to calculate the perimeter of each shape, which improves code modularity and readability. It also handles input validation, ensuring that the user enters appropriate values for dimensions and displays error messages for invalid choices.
Input /Output
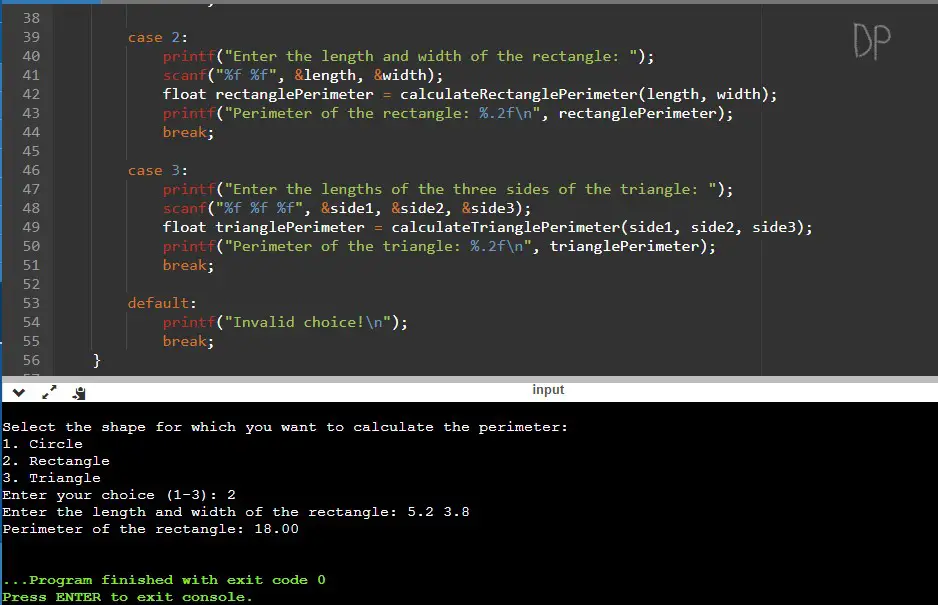
In this example, the program first displays the menu and prompts the user for their choice. The user selects option 2 (rectangle) and enters the length as 5.2 and width as 3.8. The program calculates the perimeter of the rectangle and displays it as 18.00.