In this example, we’ll create a simple C# program to determine the largest of two given numbers, demonstrating basic comparison operations.
Problem Statement
Write a C# program to find and display the largest of two numbers. The program should take two numeric inputs and determine which one is greater, then display the result as the largest number.
C# Program to Find the Largest of Two Numbers
using System; class Program { static void Main() { Console.Write("Enter the first number: "); double num1 = Convert.ToDouble(Console.ReadLine()); Console.Write("Enter the second number: "); double num2 = Convert.ToDouble(Console.ReadLine()); double largest = FindLargest(num1, num2); Console.WriteLine("The largest number is: " + largest); } static double FindLargest(double num1, double num2) { return num1 > num2 ? num1 : num2; } }
Input / Output
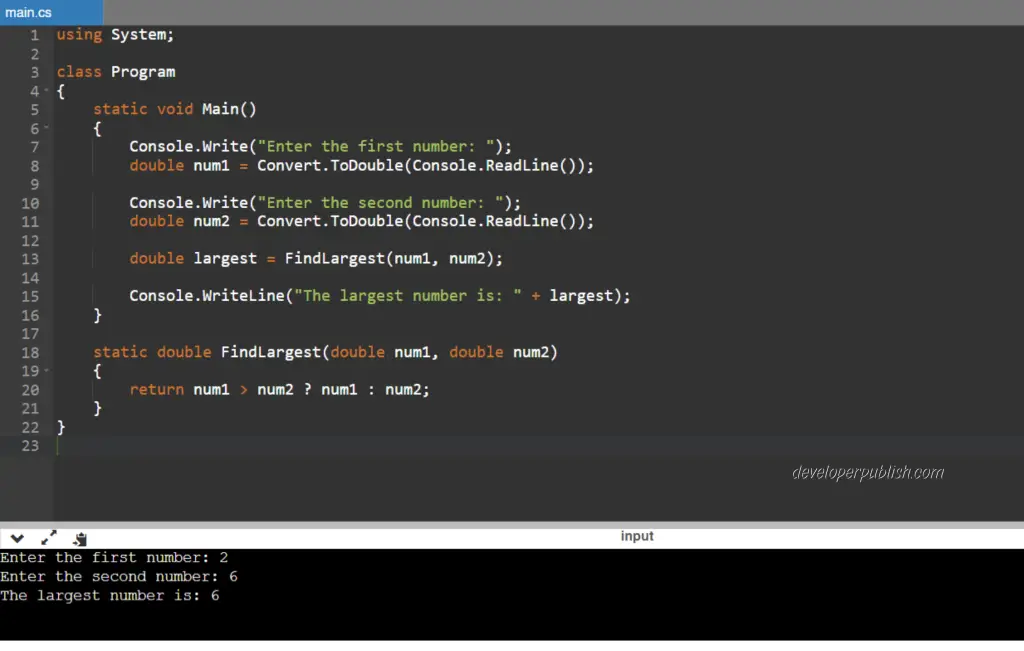