This program utilizes the C# language to calculate the average of an array of numbers. It employs fundamental concepts of array manipulation and basic arithmetic operations.
Problem statement
You are tasked with writing a C# program that calculates the average of a set of numbers stored in an array.
C# Program to Find the Average of an Array
using System; class Program { static void Main() { // Define an array of numbers int[] numbers = { 1, 2, 3, 4, 5 }; // Call the method to calculate the average double average = CalculateAverage(numbers); // Print the average Console.WriteLine("The average is: " + average); } static double CalculateAverage(int[] arr) { // Initialize sum to 0 int sum = 0; // Iterate through the array and add each element to the sum foreach (int num in arr) { sum += num; } // Calculate the average double average = (double)sum / arr.Length; return average; } }
How it works
Here’s the program with explanations:
Define an Array:
- We start by defining an integer array named
numbers
. In this example, we have hardcoded the values {1, 2, 3, 4, 5} into the array.
2. Call the CalculateAverage
Method:
- We call a method named
CalculateAverage
and pass thenumbers
array as an argument. This method is responsible for finding the average.
3. CalculateAverage Method:
- This method takes an integer array
arr
as a parameter. - It initializes a variable
sum
to 0, which will be used to accumulate the sum of all elements in the array.
4. Iterate Through the Array:
- The program uses a
foreach
loop to iterate through each elementnum
in thearr
array. - Inside the loop, it adds the value of
num
to thesum
variable, effectively summing up all the elements in the array.
5. Calculate the Average:
- After the loop has iterated through all elements, the program calculates the average. It divides the
sum
by the length of the array, which is accessed usingarr.Length
. - To ensure that the result is a floating-point number (decimal), it casts the
sum
todouble
.
6. Print the Average:
- Finally, the program prints the calculated average to the console using
Console.WriteLine
.
When you run this program, it will calculate the average of the numbers in the numbers
array and display it as output. You can customize this program by providing a different array or by accepting user input to populate the array dynamically.
Input/Output
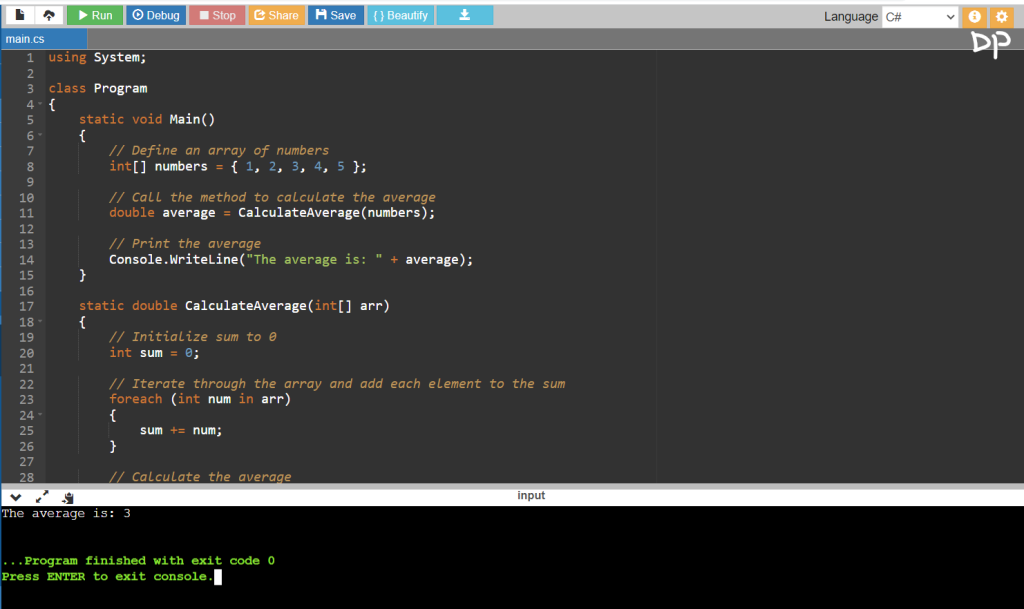