This C program calculates the sum of the first N natural numbers using a for
loop. It iterates from 1 to N and accumulates the sum by adding each number to the previous sum.
Problem Statement
Given a positive integer N, we need to find the sum of the first N natural numbers.
C Program to Find Sum of Digits of a Number using Recursion
#include <stdio.h> int sumOfDigits(int number); int main() { int number; printf("Enter a positive integer: "); scanf("%d", &number); int sum = sumOfDigits(number); printf("Sum of digits of %d = %d\n", number, sum); return 0; } int sumOfDigits(int number) { if (number == 0) { return 0; } else { return (number % 10) + sumOfDigits(number / 10); } }
How it Works:
- The user is prompted to enter a positive integer N.
- The
for
loop is used to iterate from 1 to N. The loop variablei
is initialized to 1, and the loop continues as long asi
is less than or equal to N. After each iteration,i
is incremented by 1. - Inside the loop, the current value of
i
is added to thesum
variable. This accumulates the sum of the numbers from 1 to N. - After the loop completes, the final sum is stored in the
sum
variable. - The main function displays the calculated sum on the screen.
Input/Output:
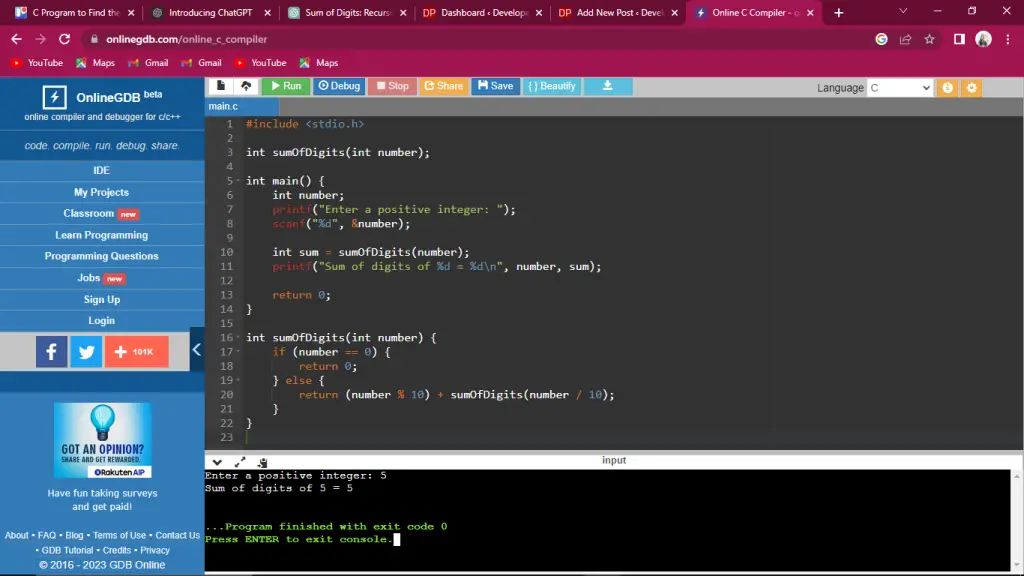