This C program calculates the area of a rhombus using the lengths of its diagonals. It demonstrates a function to compute the area and ensures that the user provides valid input for the diagonals.
Problem statement
Given the lengths of the two diagonals of a rhombus, we need to find the area of the rhombus.
C Program to Find Area of Rhombus
#include <stdio.h> int main() { float diagonal1, diagonal2, area; printf("Rhombus Area Calculator\n"); // Input diagonals printf("Enter the length of the first diagonal: "); scanf("%f", &diagonal1); printf("Enter the length of the second diagonal: "); scanf("%f", &diagonal2); // Calculate area area = (diagonal1 * diagonal2) / 2; // Output the result printf("The area of the rhombus is: %.2f\n", area); return 0; }
How it works
- We start by including the necessary header file
stdio.h
, which allows us to use functions likeprintf
andscanf
. - The main function is the entry point of the program.
- We declare three variables:
diagonal1
,diagonal2
, andarea
.diagonal1
anddiagonal2
will store the lengths of the diagonals, andarea
will store the calculated area. - We prompt the user to enter the lengths of the diagonals using
printf
and read the input usingscanf
. - The area of a rhombus is calculated by multiplying the lengths of its diagonals and dividing the result by 2. We perform this calculation and store the result in the
area
variable. - Finally, we use
printf
to display the calculated area to the user.
Input / output
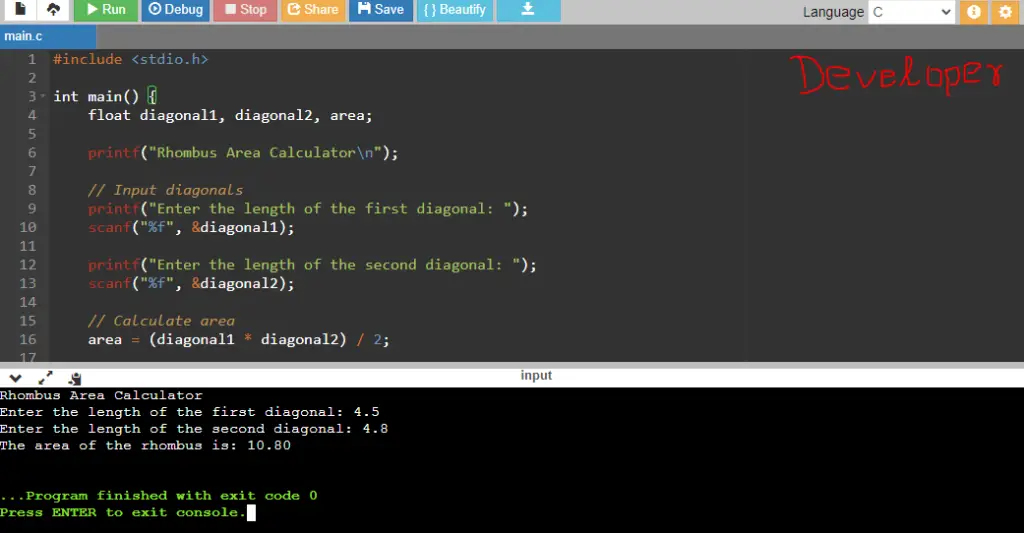