In linear algebra, a lower triangular matrix is a square matrix in which all the elements above the main diagonal are zero. In this C# program, we will generate and display a lower triangular matrix.
Problem Statement:
Write a C# program that takes an integer ‘n’ as input and generates a lower triangular matrix of size ‘n x n’ with random values for the elements below or on the main diagonal. Then, it will display the matrix.
C# Program to Display Lower Triangular Matrix
using System; class LowerTriangularMatrix { static void Main() { Console.WriteLine("Lower Triangular Matrix Generator"); Console.Write("Enter the size of the matrix (n): "); int n = int.Parse(Console.ReadLine()); int[,] matrix = GenerateLowerTriangularMatrix(n); Console.WriteLine("\nLower Triangular Matrix:"); DisplayMatrix(matrix); } static int[,] GenerateLowerTriangularMatrix(int n) { int[,] matrix = new int[n, n]; Random rand = new Random(); for (int i = 0; i < n; i++) { for (int j = 0; j <= i; j++) { matrix[i, j] = rand.Next(1, 101); // Fill with random values (1-100) } } return matrix; } static void DisplayMatrix(int[,] matrix) { int n = matrix.GetLength(0); for (int i = 0; i < n; i++) { for (int j = 0; j < n; j++) { Console.Write(matrix[i, j] + "\t"); } Console.WriteLine(); } } }
How it Works:
- The program starts by taking input for the size of the matrix ‘n’.
- The
GenerateLowerTriangularMatrix
function generates a lower triangular matrix of size ‘n x n’ with random values below or on the main diagonal. - The
DisplayMatrix
function is used to display the generated matrix. - The generated lower triangular matrix is displayed on the console.
Input/Output:
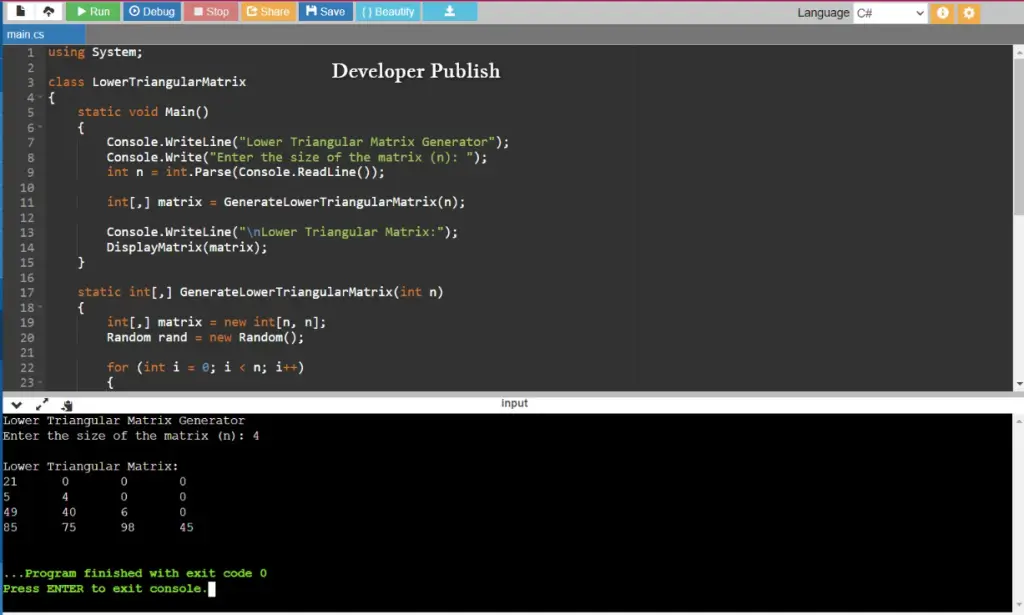